Website speed is crucial in 2025. Search engines weigh page load times in their algorithms, and users expect instant content rendering on everything from phones to desktops. Whether you’re building a small personal site or a massive e-commerce platform, these 10 performance tips can help you optimize front-end code, cut overhead, and deliver a snappy experience that pleases visitors and search bots alike.
1. Code Splitting with Bundlers
1.1 Why Code Split?
- Definition: Code splitting divides your JavaScript into smaller chunks so browsers load only what’s necessary.
- Benefits: If your entire app is in one huge
.js
file, users wait for the entire bundle—even if they only need a part of it. Splitting speeds up initial loads.
Implementation:
- Webpack: Use dynamic
import()
or configuration forSplitChunksPlugin
. - Rollup/Vite: Usually built-in code splitting for dynamic modules.
- React: Tools like React.lazy or Next.js automatically handle chunking per route/component.
Outcome: By lazy-loading modules only for relevant pages, you reduce the main bundle size, letting users see essential content faster.
2. Lazy Loading Images
2.1 The Problem
- Images can be the heaviest assets on a web page. Loading them all up front wastes bandwidth if some images remain offscreen.
- User Experience: Visitors might see a blank screen while large images are fetched.
2.2 Implementation Approaches
- Native Lazy Load: Modern browsers support
loading="lazy"
in<img>
tags. - JS Libraries: Intersection Observer–based solutions for older browsers or advanced scenarios (fade-ins, placeholders).
Example (HTML5):
htmlCopyEdit<img src="big-image.jpg" loading="lazy" alt="Lazy loading example" />
Tip: Ensure meaningful placeholders or small blur thumbnails to avoid layout shifts when images appear.
3. Minify & Compress Assets
3.1 Minifying JS and CSS
- What It Means: Removing whitespace, renaming variables, eliminating comments, e.g., with Terser (JS) or cssnano.
- Tools: Build pipelines (Webpack, Parcel, Vite) usually minify by default in production mode.
Outcome: Smaller file sizes mean quicker downloads for each script and style sheet.
3.2 GZIP or Brotli Compression
- Server-Side: Ensure your server (NGINX, Apache, or cloud config) sends compressed responses.
- Results: Dramatically reduces textual payloads—HTML, CSS, JS—by up to 70%.
- Check: Tools like
curl -I --compressed
or browser dev tools to confirm compression headers (Content-Encoding: gzip
orbr
).
4. Image Optimization & Next-Gen Formats
4.1 Use Next-Gen Formats
- WebP or AVIF: Typically smaller than JPEG/PNG while maintaining quality.
- Automatic Conversion: Tools like
sharp
or online services (like Cloudinary) to convert images on upload.
4.2 Resize & Quality Tuning
- Right-Sized: Don’t upload a 4000px wide image for a 500px display.
- Quality: For JPEG, a quality setting around 70% often gives a good trade-off. For WebP, you can tweak compression parameters further.
Pro Tip: If you want further control, consider a build script or an image CDN that dynamically serves smaller images for mobile screens.
5. Caching & Content Delivery Networks (CDNs)
5.1 Browser Caching
- Cache-Control Headers: By specifying
max-age
orimmutable
, you let browsers store assets locally for repeated visits. - Versioning: For updated files, use hashed filenames (e.g.,
bundle.abc123.js
) so old versions remain cached without messing up new releases.
5.2 CDNs for Global Reach
- Why: A CDN ensures users fetch static content from a server physically close to them, lowering latency.
- Integration: Popular CDNs (Cloudflare, AWS CloudFront, etc.) can handle your images, JS, or even entire site.
Outcome: Minimizes round-trip times, especially for users in far-flung regions.
6. Prioritize Above-the-Fold Content
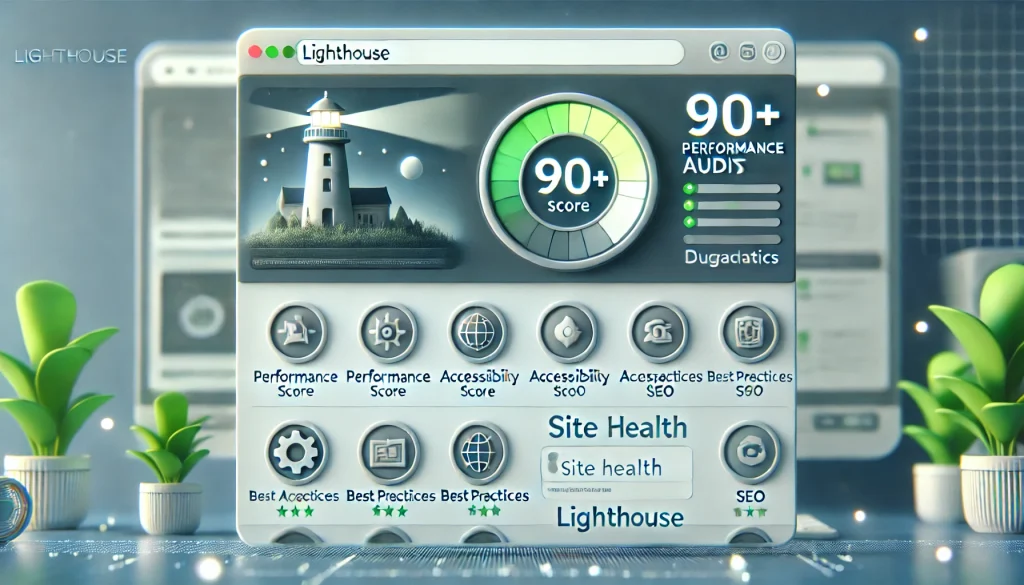
6.1 Critical CSS
- Inline the minimal CSS needed for the top of the page. Defer the rest.
- Focus: This ensures the first screenful (header, hero section, nav) loads instantaneously, even if deeper style sheets parse later.
6.2 Defer Non-Critical Scripts
- Attribute:
defer
orasync
on<script>
to keep them from blocking initial rendering. - Splitting: For optional features or analytics, load them after main content is displayed.
Tip: Tools like Lighthouse
can highlight which scripts hamper first paint. Defer them if not essential for immediate user interaction.
7. Code Cleanup & Removing Unused Libraries
7.1 Tree Shaking
- ES Modules: Many build tools can “tree shake” unused exports if you import only what’s needed.
- Third-Party Bloat: A single large library can overshadow the rest of your code. Evaluate if a small utility or manual snippet suffices.
7.2 Auditing Dependencies
- NPM scripts: Check
npm ls --prod
to see your production dependencies, or use a tool likewebpack-bundle-analyzer
to visualize chunk sizes. - Refactoring: If you find you only use a fraction of a big library, consider a smaller alternative or direct utility function.
Outcome: Leaner bundles reduce network overhead, especially on mobile or lower-speed networks.
8. Common Free Tools for Testing Performance
- Google Lighthouse: Built into Chrome DevTools, measures page speed, SEO, accessibility, best practices.
- PageSpeed Insights: Google’s online version, plus suggestions for improvements.
- GTmetrix: Another well-known site performance analyzer, offering historical comparisons.
- WebPageTest: Detailed waterfall charts, simulating real user conditions.
- Pingdom: Basic performance insights plus uptime monitoring.
Use: Focus on metrics like Largest Contentful Paint (LCP), First Input Delay (FID), and Cumulative Layout Shift (CLS) to gauge real user experiences.
9. Lazy Loading Other Resources (Videos, Scripts)
9.1 Video Loading
- Poster: Provide a static poster image to avoid auto-loading the entire video.
- Intersection Observer: Start loading or playing the video only when it’s close to the viewport.
- Format: Consider streaming solutions or lighter MP4 compressions for short clips.
9.2 Third-Party Scripts
- Async or Defer: If you embed external analytics or ads, ensure they don’t block your main thread.
- Tag Manager: Tools like Google Tag Manager can centralize third-party scripts, letting you control or postpone them as needed.
10. Combining Gzip/Brotli with SSR
10.1 Server-Side Rendering (SSR)
- Framework: Next.js, Nuxt, SvelteKit, or custom Node setups let you deliver HTML pre-rendered, speeding up initial load.
- Client-Side: Then rehydrate with JS, so the page remains dynamic.
10.2 Gzip or Brotli on SSR Output
- HTTP Headers: By compressing SSR’d HTML, you minimize payload size for that crucial first impression.
- Example: Nginx config: nginxCopyEdit
gzip on; gzip_types text/html text/css application/javascript;
Outcome: Coupling SSR with compression yields near-instant content, especially beneficial for content-heavy sites.
Conclusion
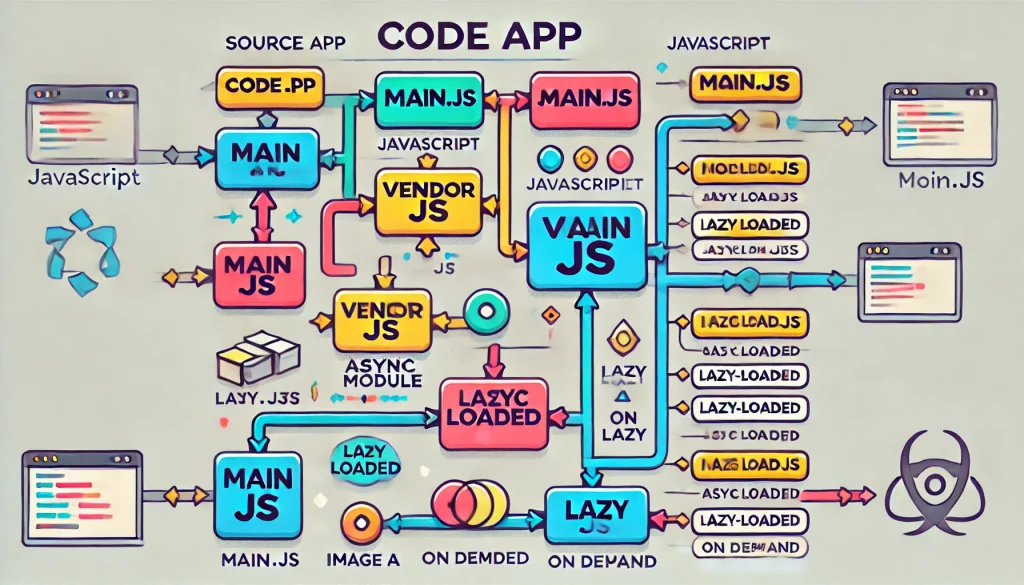
Optimizing front-end performance can be simple or complex depending on your site’s architecture. But with these 10 tips—ranging from code splitting and lazy loading to CDN usage and minifying assets—you’ll ensure your site loads fast and ranks better in SEO while pleasing visitors. Combine free testing tools (Lighthouse, GTmetrix, PageSpeed) to highlight further bottlenecks and confirm your improvements. Through consistent iteration, you’ll master the art of a blazing-fast user experience in modern web development.