Regular expressions—commonly called regex—are a powerful way to search, match, and manipulate text. Yet despite its utility, many developers find regex tricky to remember or decipher. Whether you’re validating an email, splitting log files, or performing a quick find-and-replace, having a quick reference guide can be transformative. Below is an overview of core regex tokens, quantifiers, capturing groups, plus real-world usage examples to boost your regex confidence.
1. Why Regex Matters
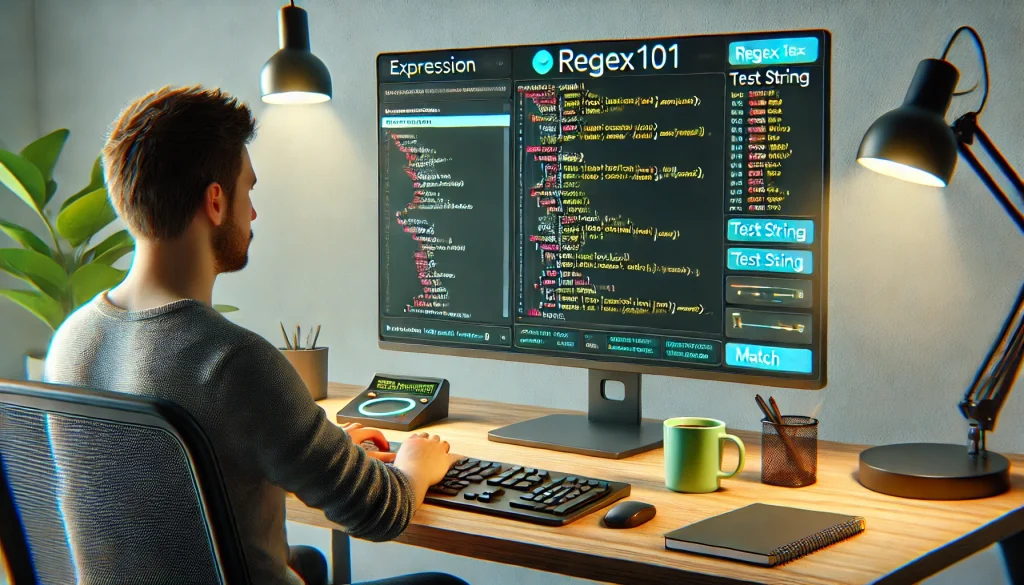
- Versatility: From searching plain text to extracting data from complicated logs, regex can handle structured or semi-structured patterns quickly.
- Universal Support: Most programming languages (Python, JavaScript, Java, etc.) have built-in or library support for regex.
- Efficiency: Instead of writing multiple lines of code to parse strings, a well-crafted regex can do it in a single expression—once you master the syntax!
Challenge: The same powerful syntax that makes regex so flexible can appear cryptic. Understanding it is key to harnessing that power.
2. Regex Quick Reference Cheat Sheet
2.1 Common Special Characters
Symbol | Meaning | Example |
---|---|---|
. | Matches any character (except newline) | a.c matches "abc" , "adc" |
\d | Digit (0–9) | \d\d finds any two consecutive digits |
\w | Word character (letters, digits, underscore) | \w+ matches a string of word chars |
\s | Whitespace (spaces, tabs, newlines) | \s+ matches multiple whitespace chars |
^ | Start of string (or line in multiline mode) | ^Hello matches lines starting with "Hello" |
$ | End of string (or line in multiline mode) | end$ matches lines ending in "end" |
[...] | Character class | [aeiou] matches any vowel |
[^...] | Negated character class | [^0-9] matches non-digit characters |
2.2 Quantifiers
Quantifier | Description | Example |
---|---|---|
* | Matches 0 or more occurrences | a* = "" (empty), "a", "aa", "aaa", ... |
+ | Matches 1 or more occurrences | a+ = "a", "aa", "aaa", ... |
? | Matches 0 or 1 occurrence | colou?r = matches "color" or "colour" |
{n} | Matches exactly n occurrences | \d{3} = exactly 3 digits, e.g. "123" |
{n,} | Matches at least n occurrences | \w{5,} = 5 or more word characters |
{n,m} | Matches between n and m occurrences | [A-Z]{2,4} = between 2 and 4 uppercase letters |
2.3 Capturing & Grouping
- Capturing Groups:
( ... )
- Allows retrieval of matched sub-patterns.
- Example:
(\d{3})-(\d{4})
can capture two groups (like123-4567
), letting you reference them as group 1, group 2 in your code.
- Non-Capturing Groups:
(?: ... )
- Groups multiple tokens but won’t store them as a captured group.
- Useful for grouping quantifiers or applying a quantifier to multiple tokens but skipping overhead of capturing.
- Backreferences:
\1
,\2
- Reuse matched text from a capturing group later in the pattern.
- Example:
(\w)\1
matches any two identical word characters in a row, e.g."ee"
in"coffee"
.
3. Real-World Regex Examples
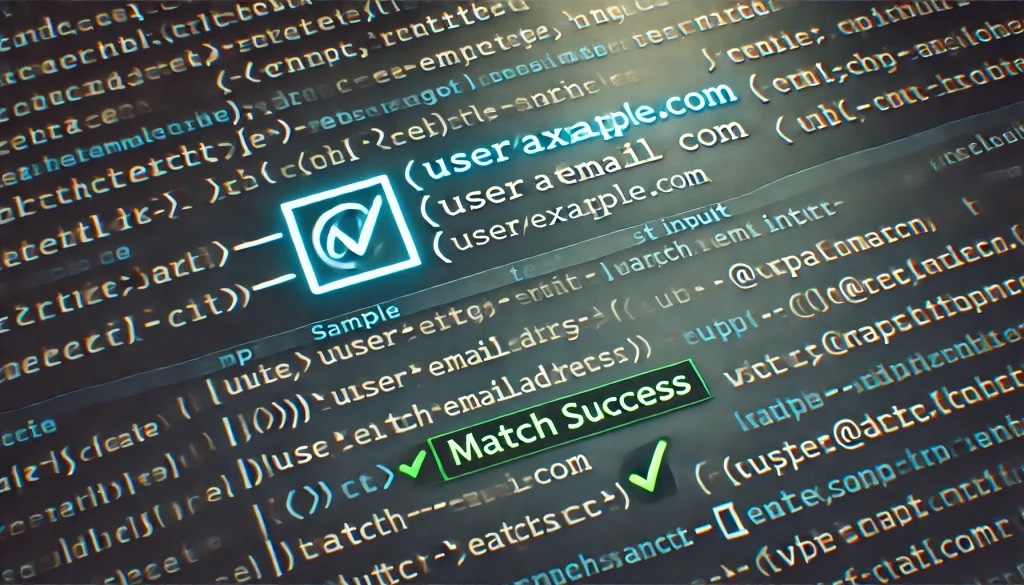
3.1 Validating an Email
Regex:
scssCopyEdit^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$
Explanation:
^[A-Za-z0-9._%+-]+
ensures the email’s local part (before@
) has letters, digits, or select punctuation.@[A-Za-z0-9.-]+
matches the domain name (letters, digits,.
,-
).\.[A-Za-z]{2,}$
requires a top-level domain of at least 2 letters.^...$
means it must match the entire string, not just a substring.
Tip: Email validation can get more complex, but this pattern is a decent start. If your domain’s TLD can be longer (like .solutions
), ensure it can handle more characters (3, 4+).
3.2 Matching a US Phone Number Format
Regex:
rubyCopyEdit^\(\d{3}\)\s?\d{3}-\d{4}$
Explanation:
^\(
requires an open parenthesis at start.\d{3}
matches exactly 3 digits.\)\s?
checks for a closing parenthesis plus optional space.\d{3}-\d{4}
ensures a format like123-4567
for the rest of the number.$
enforces end of string.
Note: For Aussie phone numbers or other region-specific formats, the pattern changes. For instance, [0-9]{10}
or more elaborate groupings.
3.3 Splitting a Log File by Timestamp
Regex (example snippet for a split
in code):
cssCopyEdit^\d{4}-\d{2}-\d{2}\s\d{2}:\d{2}:\d{2}
Explanation:
- Matches lines starting with a date/time stamp:
YYYY-MM-DD hh:mm:ss
. - Used in multiline logs to identify where a new log entry begins if you parse them line by line.
Consider: If you handle multiline logs, you might use multiline or singleline modes and anchors accordingly.
4. Best Practices & Pitfalls
4.1 Avoid Over-Complex Patterns
- Readability: Some monstrous one-liner might “work,” but it becomes unmaintainable.
- Performance: Overly nested patterns or catastrophic backtracking can degrade performance drastically.
Pro Tip: Break long patterns into smaller sub-patterns or use comments and verbose mode (where supported by your language).
4.2 Test Thoroughly
- Online Tools: Use sites like regex101 or regexr to debug and confirm group captures.
- Edge Cases: Test with empty strings, unusual input (accents?), or partial matches.
- Runtime: If used in user-facing code, ensure it handles large input or concurrency without hogging resources.
4.3 Keep Security in Mind
- User Input: Always sanitize or limit user-provided regex patterns to avoid ReDoS (Regular Expression Denial of Service).
- Validation: Regex can validate formats but can’t ensure data is logically correct (like ensuring a domain is real or a phone number is active).
5. Additional Resources
- Official Docs: Most languages have a “RegExp” reference or library-specific doc (e.g.,
re
in Python,java.util.regex
, JavaScript’sRegExp
). - Regex Cheat Sheets: Keep a personal cheat sheet for quick copy-paste of common patterns.
- Community: Stack Overflow or Slack channels can quickly help debug complicated patterns or edge use-cases.
Conclusion
Mastering regex doesn’t require memorizing every symbol—just a solid grasp of the fundamentals: tokens, quantifiers, capturing groups, plus a reference for more advanced features. By learning from real-world patterns like email validation or phone formatting, you can apply these text-manipulation superpowers to any project, from simple search scripts to robust data validations. Coupled with consistent testing and attention to performance, regex can be a potent ally in your developer toolbox.