Debugging is an essential skill for any developer—no matter the language, framework, or domain. It’s often where devs spend much of their time, chasing elusive bugs or performance bottlenecks. Below are 15 crucial techniques and tools that can help you systematically find and fix issues, boosting productivity and reducing frustration along the way.
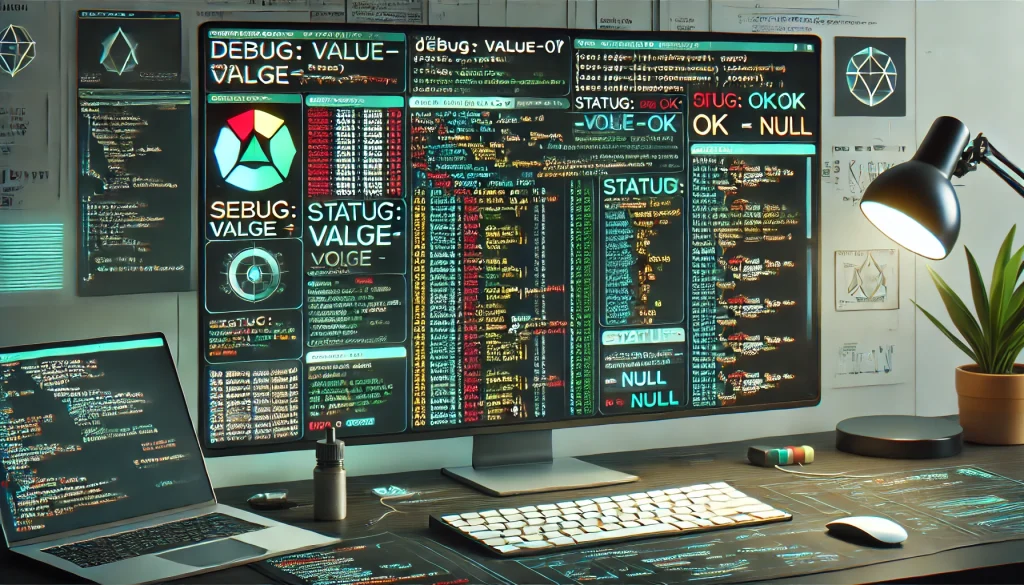
1. Classic Print (or Log) Debugging
Why It’s Useful
- Universally Available: Any language or environment can output logs or print statements.
- Simplicity: Inserting
console.log
,print()
, or log statements can quickly show variable values or function flow.
Tip: Keep logs organized—use timestamps, log levels, or unique tags. Remove or reduce them in production to avoid clutter or performance overhead.
2. Using Breakpoints & Interactive Debuggers
Why It’s Important
- Step-by-Step: Halt code execution at specific lines, then inspect variable states or call stacks in an IDE debugger.
- Conditional Breakpoints: Debuggers let you break only if certain conditions are met (e.g.,
i == 100
).
Popular Tools:
- Visual Studio Code Debugger
- IntelliJ IDEA / PyCharm integrated debuggers
- Chrome DevTools for front-end debugging
3. Binary Search (Bug Bisecting)
Why It’s Effective
- Narrow Down: If you know a certain revision or code path introduced a bug, do a “bisect” to find the offending change quickly.
- Git Bisect: Automates testing each commit in half-interval steps until it isolates the commit that caused the failure.
Key Insight: Great for large codebases or when the bug emerges between known good and bad versions.
4. Logging Frameworks & Structured Logs
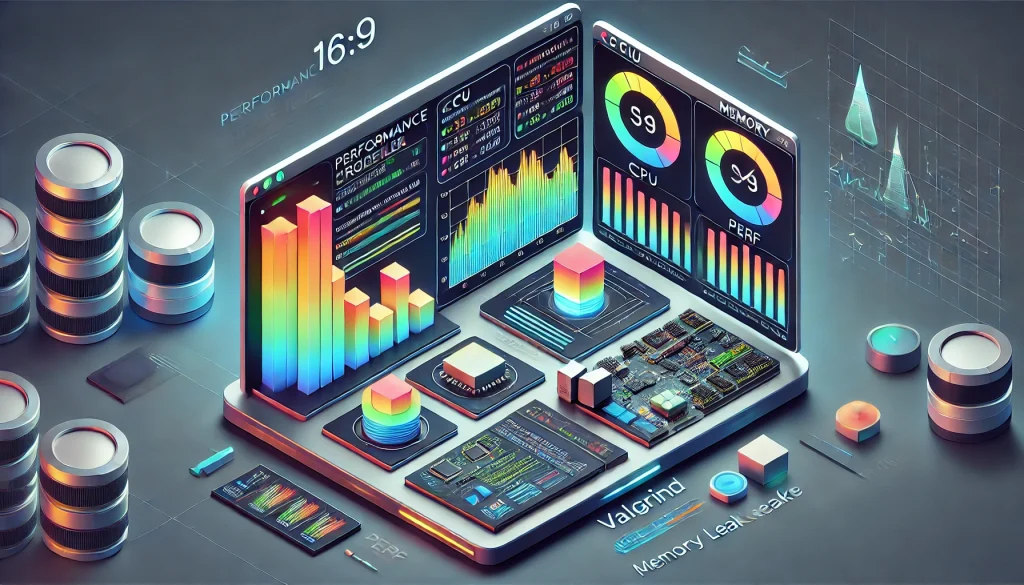
Why It’s Powerful
- Readable, Searchable Logs: Tools like Log4j (Java), Winston (Node.js), or
logging
(Python) let you standardize message formats and levels (INFO, WARN, ERROR). - JSON / Structured: Structured logs are easier for searching in centralized solutions (e.g., ELK stack, Splunk).
Outcome: Quicker triage when an error arises in production logs, especially at scale or in microservice architectures.
5. Profiling Tools (Perf, Profiler, etc.)
Why It’s Important
- Identify Performance Hotspots: Tools like Linux
perf
, Windows Performance Analyzer, or built-in language profilers (e.g., Python’s cProfile) reveal which functions or lines hog CPU time. - Memory Profiling: Check allocations, detect memory leaks with Valgrind’s
memcheck
or .NET CLR Profiler.
Use Cases: Before optimizing code, gather data from a profiler to ensure you’re tackling the biggest bottlenecks rather than guesswork.
6. Valgrind for Memory/Trace Analysis
Why It Stands Out
- C/C++ Memory: Valgrind’s
memcheck
detects invalid reads/writes, memory leaks, uninitialized variables. - Callgrind & KCachegrind: Additional tools track function call frequencies, cycles, or performance hotspots.
Example: Perfect for debugging segmentation faults or weird crashes in lower-level languages.
7. Sys Internals & OS Tools
Why These Matter
- Windows Sysinternals: Tools like Process Explorer, Process Monitor help watch system calls, registry usage, file I/O.
- Linux Tools:
strace
for system calls,ltrace
for library calls,dmesg
for kernel messages. - macOS Instruments: Observes CPU, memory, disk usage in real-time with advanced analysis.
Outcome: Understand your app’s environment-level interactions, quickly unveiling file permission or system resource issues.
8. Remote Debugging & Live Patching
Why It’s Handy
- Production Diagnostics: Attach a debugger to a running service in staging or production (with caution) to watch variables, log partial info, or inject code patches.
- Containerized Envs: Docker or Kubernetes ephemeral pods might need remote attach features for thorough debugging.
Warning: Always limit remote debugging on production systems—set authentication and minimize performance overhead or risk.
9. Unit Tests for Regression
Why It’s Foundational
- Preemptive Bug Detection: Write or expand tests once you fix an issue, ensuring it never reappears.
- Confidence: If test coverage is high, you can safely refactor or unify code flows without introducing new bugs.
Best Practice: For each discovered bug, create a failing test that replicates it, then confirm the fix passes.
10. Memory Dumps & Crash Analysis
Why It Helps
- Post-Mortem: If your app crashes in production, generating a core dump or memory dump can reveal the state of variables, call stacks, or corruption at the crash moment.
- Symbols & Debug Info: Tools like WinDbg or GDB can read symbolic info to pinpoint the function or line at fault.
Pro Tip: Keep your debug symbols for each build so you can interpret dumps months later if an old version fails.
11. Logging in External Systems (ELK Stack, etc.)
Why It’s Critical
- Centralization: Shipping logs to Elasticsearch + Logstash + Kibana (ELK) or Splunk means you can correlate events across microservices.
- Alerts: Real-time triggers when repeated errors or unusual patterns spike, helping you discover silent issues quickly.
Scenario: Complex distributed systems where cross-service correlation is the only way to see the bigger picture.
12. Time-Travel Debugging
Why It’s Cutting-Edge
- Record & Replay: Tools like Mozilla’s RR or Chronon let you “rewind” a program’s execution, stepping backward in the debugger.
- Elusive Bug Reproduction: Perfect for heisenbugs or concurrency errors that vanish when you add print statements.
Downsides: Often overhead is high, but it can be a lifesaver for concurrency or timing-based issues.
13. Concurrency Debuggers & Race Detectors
Why They’re Unique
- Thread Sanitizer (TSan): In Clang/GCC for C/C++, identifies race conditions.
- Data Race Tools: Tools for Java (like Java Flight Recorder) or .NET concurrency analyzers.
- Lock & Wait Graph: Some debuggers show lock acquisition order for deadlock detection.
Crucial for multi-threaded or parallel applications, especially HPC or real-time systems where scheduling can hide deep concurrency bugs.
14. Simulation Environments or Emulators
Why They’re Handy
- Hardware-Specific: Mobile devs rely on iOS/Android simulators, or embedded devs use QEMU for CPU architecture testing.
- Network Simulation: Tools like Mininet for simulating large networks can reveal race conditions or resource conflicts not visible on a local dev machine.
Scenario: Checking performance, resource usage, or fault tolerance in an environment approximating the real hardware or network environment.
15. Pair Debugging & Rubber Duck
Why They Still Matter
- Pair Programming: Another dev might notice a subtle assumption you missed.
- Rubber Duck Debugging: Explaining the problem aloud, even to an inanimate object, clarifies your logic and helps you see mistakes.
Practical: Sometimes the simplest debugging approach is stepping away from the code and describing it to a colleague or imaginary audience to find the glitch.
Conclusion
Mastering debugging goes beyond a single tool or approach. From basic print statements and breakpoints to sophisticated concurrency analyzers and time-travel debuggers, each technique suits a certain class of issues. By building a diverse debugging toolkit and leveraging the right approach at the right time, you can unravel even the most elusive bugs, protecting your software’s stability and performance.
Key Takeaways:
- Start simple: print/log-based debugging reveals the immediate flow and variable states.
- Embrace advanced profilers, memory analyzers, concurrency checkers, and post-mortem tools for more complex issues.
- Use tests, version control bisects, and multi-level logging to prevent reoccurrences.
- Don’t overlook pair debugging or rubber duck methods—they often solve logic flaws quickly.
Whether you’re building kernel modules in C or writing JavaScript for the web, these 15 debugging techniques provide a solid foundation for tackling errors swiftly and systematically—reducing dev headaches and shipping robust code with confidence.