Introduction
GitHub is a powerful platform for hosting code, collaborating with others, and showcasing your projects. For entry-level developers, the initial steps—creating a repository, setting up Git, and handling the first push—can be confusing. This tutorial will guide you from zero to having your code publicly (or privately) available on GitHub.
By the end, you’ll know how to:
- Create a GitHub repo.
- Configure Git and link your local project.
- Stage, commit, and push your code.
- Write a simple README to introduce your project.
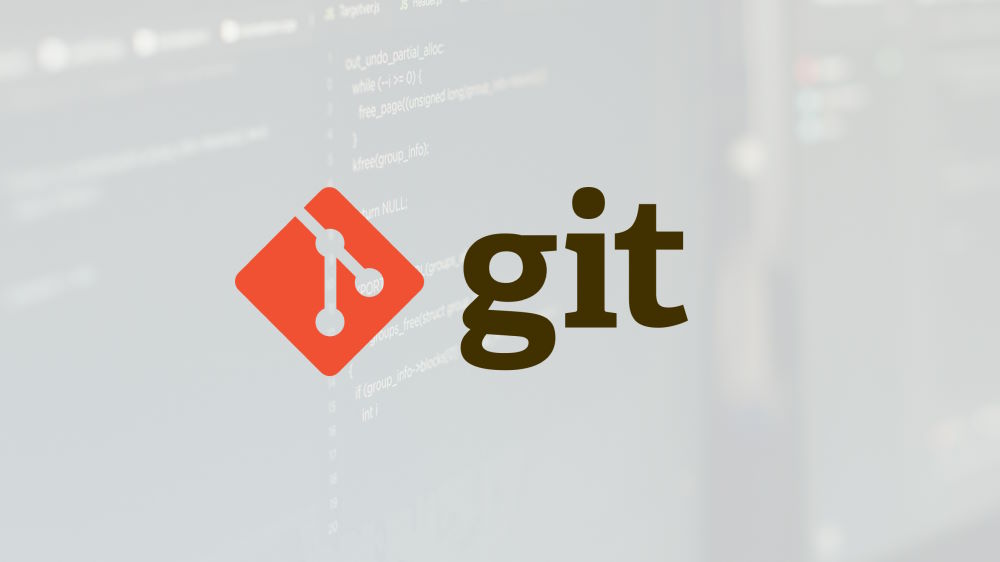
1. Prerequisites
Before you begin, make sure you have:
- Git installed: Download Git for your OS.
- GitHub account: Sign up for GitHub if you haven’t.
- Local project folder: Have some starter code or at least a placeholder file.
2. Setting Up SSH (Optional But Recommended)
You can connect to GitHub via HTTPS or SSH. SSH is often preferred because it’s more secure and won’t prompt you for credentials every time.
2.1 Generating an SSH Key
- Open Terminal / Git Bash:
- On Windows, you can use Git Bash.
- Run: bashCopyEdit
ssh-keygen -t ed25519 -C "[email protected]"
- Save the generated key files (
id_ed25519
andid_ed25519.pub
) in the default location or a secure place you’ll remember. - No passphrase?: You can leave it empty or add one for extra security.
2.2 Adding Your SSH Key to GitHub
- Copy the contents of your .pub file: bashCopyEdit
cat ~/.ssh/id_ed25519.pub
- Go to GitHub → Click your profile → Settings → SSH and GPG keys → New SSH key.
- Paste the public key in the Key field and give it a title.
- Click Add SSH key.
3. Creating a GitHub Repository
- Sign in to GitHub.
- Click the green “New” or “+” icon to create a new repository.
- Name your repository: Choose something descriptive like
my-first-repo
. - Decide if you want it public or private.
- (Optional) Initialize with a README if you’d like GitHub to create one for you.
- Click Create Repository.
You’ll land on a page with instructions for connecting your local project to this new repo.
4. Initializing Your Local Project
If you don’t already have a .git folder in your local project, you need to initialize Git:
- Open terminal and navigate to your project folder: bashCopyEdit
cd path/to/your/project
- Initialize: bashCopyEdit
git init
This command creates a hidden .git
directory, turning your folder into a Git repository.
5. Linking Local Code to GitHub
5.1 Adding the Remote
- Grab the repository URL from GitHub. If you’re using SSH, it typically looks like: perlCopyEdit
[email protected]:username/my-first-repo.git
- Link your local repo to GitHub: bashCopyEdit
git remote add origin [email protected]:username/my-first-repo.git
origin
is a conventional alias for this remote repository.
5.2 Checking Remote Connectivity
To verify the new remote:
bashCopyEditgit remote -v
This should list origin
along with the URL you just added.
6. Commit and Push Code
6.1 Stage and Commit
- Stage all changes: bashCopyEdit
git add .
This adds every new or modified file to the staging area. - Commit: bashCopyEdit
git commit -m "Initial commit"
Always include a short, descriptive commit message.
6.2 Push Code to GitHub
Finally, upload your local commits to the GitHub repository:
bashCopyEditgit push -u origin master
-u
setsorigin master
as the default upstream branch, so future pushes only requiregit push
.- Some modern repos use
main
instead ofmaster
. Adjust accordingly (e.g.,git push -u origin main
).
7. Creating a Basic README
A README file helps others understand your project. Many devs also use it for personal reference.
Sample README.md:
markdownCopyEdit# My First Repo
This repository is my initial foray into GitHub. It includes a simple script that prints "Hello, world!"
## Features
- Beginner-friendly Python code
- Example usage of Git and GitHub workflows
- Placing
README.md
in your project root ensures GitHub displays it automatically on the repo page.
8. Common Issues and How to Fix Them
- Authentication Failed
- Double-check your SSH key setup or switch to HTTPS.
- Confirm that you added the correct public key to GitHub.
- Permission Denied
- Ensure your username matches your GitHub account.
- Make sure you have write access if it’s a team repository.
- Branch Name Conflicts
- Some repos default to
main
instead ofmaster
. Use the correct branch name ingit push
.
- Some repos default to
- Untracked Files
- If certain files don’t appear on GitHub, verify that you used
git add
before committing or that they’re not ignored via.gitignore
.
- If certain files don’t appear on GitHub, verify that you used
Conclusion
Pushing a project to GitHub for the first time is a major milestone for new developers. By initializing a local Git repository, setting up SSH (or HTTPS), and learning a few essential commands, you can now showcase your code to recruiters, collaborators, or the open-source community. Remember to add a helpful README, commit changes frequently, and keep exploring Git’s more advanced features like branching and pull requests.
Key Takeaways:
- Initialize Git locally with
git init
. - Link to GitHub with
git remote add
. - Stage & Commit changes, then push to share your code.
- Use a README to describe your project’s purpose and usage.
Next Steps:
Explore more Git functionality—like branching, merging, and pull requests—to collaborate effectively. Happy coding!