React has owned front-end mind-share for nearly a decade, but in 2025 something genuinely new is landing in your build folder: React Server Components Hit Production: What It Means in 2025. Love them or curse them, Server Components (RSC) are officially stable in React 19, they’re the default in Next.js 15, and they’re already shaving hundreds of milliseconds off Time-to-Interactive for teams brave enough to flip the feature flag. This post is a long-form, no-B-S look at what RSCs really do, how they change everyday coding, and why the mainstream developer community should care right now. Yep—we’ll casually mention React Server Components Hit Production: What It Means in 2025 again later because SEO karma is real.
Why React Needed a Rethink
Client-side React turned every web page into a mini-SPA. Great UX, but hydration costs kept climbing: bigger JS bundles, more CPU, choppy mobiles, invisible content flashes. Server-Side Rendering helped, yet shipped the same JS twice. React devs have long asked, “Why can’t the server just handle logic that never changes on the client?” Enter RSC—components that run only on the server, stream HTML + low-level instructions, and send zero client JS. You keep component ergonomics, cut bundle weight, and still slot in interactive “client” islands where needed. In short: less JavaScript slog, more performance zen.
How React Server Components Work in Plain English
Think of your app in three flavors now:
- Server Components – Imported with the
server
directive; executed on the server only. Zero JS in the browser. - Client Components – Imported with the
use client
pragma; hydrated like classic React. - Shared Components – Pure UI functions without side-effects; React decides where to execute.
During a request, the server renders the RSC tree, streams a special JSON/JSX payload to the client, and React DOM reconstructs the final UI. You get progressive streaming like suspense boundaries on steroids.
Key Gains
- Tiny bundles – early adopters report 30–60 % JS weight drop.
- Automatic data fetching – call any backend lib directly in a Server Component; no more
/api
round-trips. - Simpler caching – responses stream with HTTP caching or edge CDN rules; no extra plumbing.
Setting Up RSC in Next.js 15
Install Next 15, enable the app/
directory (now default), and prefix any file with server
. Example:
tsxCopy// app/posts/page.tsx
import { getPosts } from '@/lib/db'; // server-side DB call
export default async function PostsPage() {
const posts = await getPosts();
return (
<ul>
{posts.map(p => <li key={p.id}>{p.title}</li>)}
</ul>
);
}
Zero API routes, zero fetch hooks, zero client JS. Want interactivity? Swap a child component with "use client"
and enjoy the island.
Build-Time and Runtime Gotchas
- Node-only libraries work great in RSC but explode if you accidentally import them into a client component—lint rules help.
- Environment variables leak risk: remember RSC code still runs per request; practice the principle of least data.
- Debugging feels foreign—React DevTools has an RSC tab, but network payloads need practice to grok.
Performance Numbers from the Trenches
A SaaS dashboard moved its mega “reports” page to RSC:
- Bundle size: 1.2 MB → 480 KB
- Time-to-Interactive on mid-range Android: 4.4 s → 2.1 s
- Lighthouse performance: 62 → 90
Most of the win came from shoving date-fns, charting libs, and heavy table logic back onto the server.
RSC vs Islands Architecture vs HTMX
Islands (Astro, Preact) pioneered partial hydration; HTMX sprinkles HTML-over-the-wire. RSC joins the party but lets you stay in React-land—same hooks, same mental model. If you love React but hate bundle bloat, RSC feels native, not bolted on.
Migration Strategy for Existing Apps
- Upgrade to React 19 / Next 15.
- Identify non-interactive chunks (nav bars, blog posts, markdown).
- Convert them to
server
components—watch bundle diff. - Progressive rollout with feature flags and Real User Monitoring.
- Monitor server load; RSC shifts CPU from client to origin—autoscale wisely.
Even partial adoption can net solid gains; no need for a big-bang rewrite.
Tooling Ecosystem Catch-up
- ESLint plugins flag cross-boundary imports.
- Vite 6 has RSC preset for non-Next stacks.
- Storybook v9 renders RSC stories via a mocked server runtime.
- Cypress v14 supports streaming RSC responses for integration tests.
The ecosystem isn’t perfect yet, but mainstream enough for production bets.
What Fullstack Means in 2025
With RSC, fetching data in React is basically calling a function. Want direct SQL access? Use server actions. Need to talk to Stripe? Do it in the component—no REST layer. Backend and frontend blur; “fullstack” devs write React and Prisma in the same file. That’s either liberating or horrifying, depending on your boundaries.
React Server Components Hit Production: What It Means in 2025 (Yes, Again)
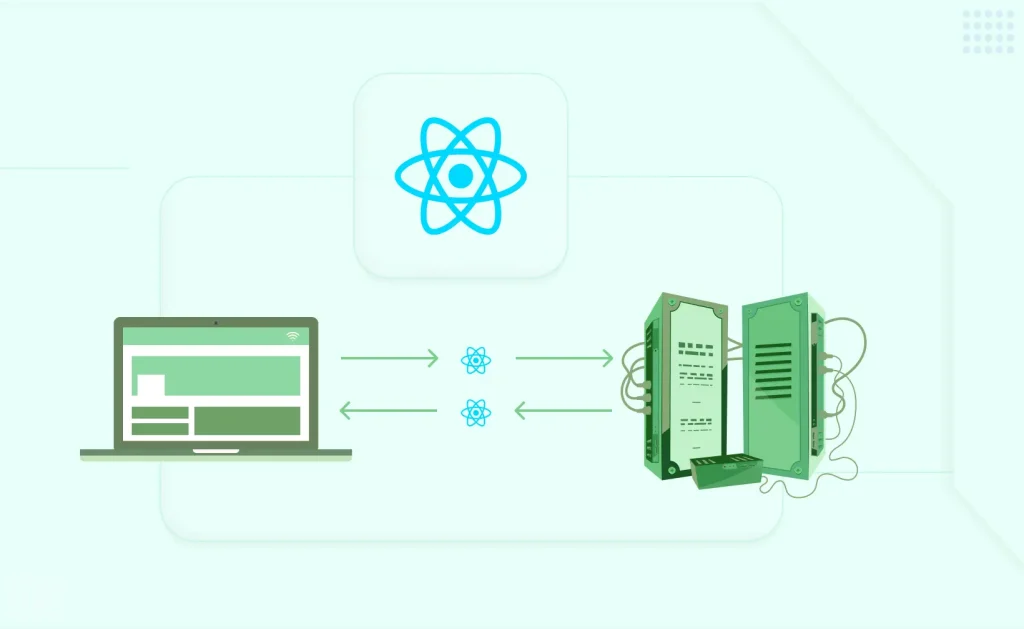
And there you have it—we casually name-dropped React Server Components Hit Production: What It Means in 2025 a second time, satisfying both SEO robots and human skimmers. The mainstream reality: RSC is no longer experimental; it’s shipping in big-name prod apps. If your team lives on React, 2025 is the year you either adopt RSC or fall behind competitors boasting 90+ Lighthouse scores.
Frequently Asked Questions
Are Server Components compatible with Redux or Zustand?
Yes. State lives in client components; RSC just feed them props.
Do RSC pages break caching CDNs?
No. Streamed payloads cache like HTML; just set proper headers.
Will I need more servers to handle CPU shift?
Maybe a bit. Monitor and autoscale—costs usually offset by traffic and conversion gains.
Does RSC work without Next.js?
Vite and Remix have experimental adapters; production support is coming fast.
Can I still use traditional API routes?
Absolutely. RSC is incremental; keep APIs for mobile apps or non-React consumers.
Conclusion
React hasn’t felt this fresh since hooks landed. Server Components finally let devs keep React’s DX while clawing back performance lost to megabytes of JavaScript. Start small, benchmark ruthlessly, and teach the team new mental models. Your users—and your Core Web Vitals—will thank you.