For full-stack developers, Visual Studio Code (VS Code) has become a go-to editor—combining a sleek interface, powerful integrations, and a huge extension library. Still, with so many plugins available, developers often wonder which ones really elevate productivity or are must-haves. Below, we’ll dive into essential VS Code extensions (like Prettier, ESLint, Live Server), show how to set up debugging, highlight integrated Git workflows, and offer tips for custom snippets. Whether you’re coding Node.js back ends, React front ends, or a bit of everything, these tools can streamline your dev environment immensely.
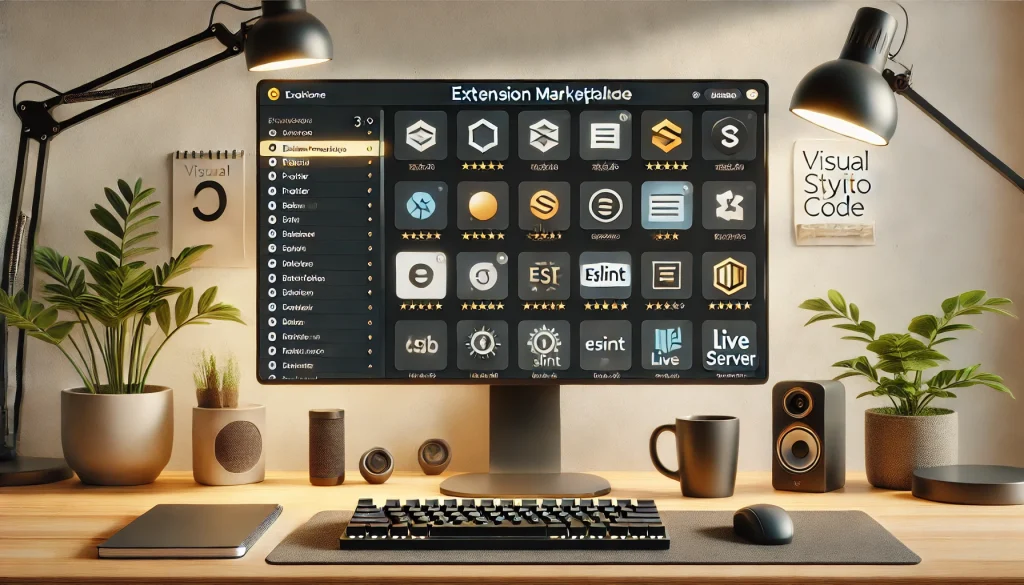
1. Why VS Code Dominates for Full-Stack Devs
1.1 Speed and Extensions
- Lightweight Core: VS Code runs smoothly on many systems, letting devs spin it up quickly.
- Marketplace: Thousands of extensions, from language support to productivity tools, adapt it for any stack—Node, Python, .NET, or front-end frameworks like React or Vue.
Outcome: Devs can unify back-end and front-end tasks in a single environment, accelerating multi-project workflows.
1.2 Built-In Git & Terminal
- Git Integration: Basic Git commands—commit, branch, push—are integrated in the sidebar, removing the need for external GUIs or separate terminal calls.
- Integrated Terminal: Switch seamlessly between code and shell commands (npm, yarn, docker, etc.) in one window.
Tip: If your project is multi-repo or microservices-based, you can open multiple folder workspaces to manage them concurrently.
2. Must-Have Extensions
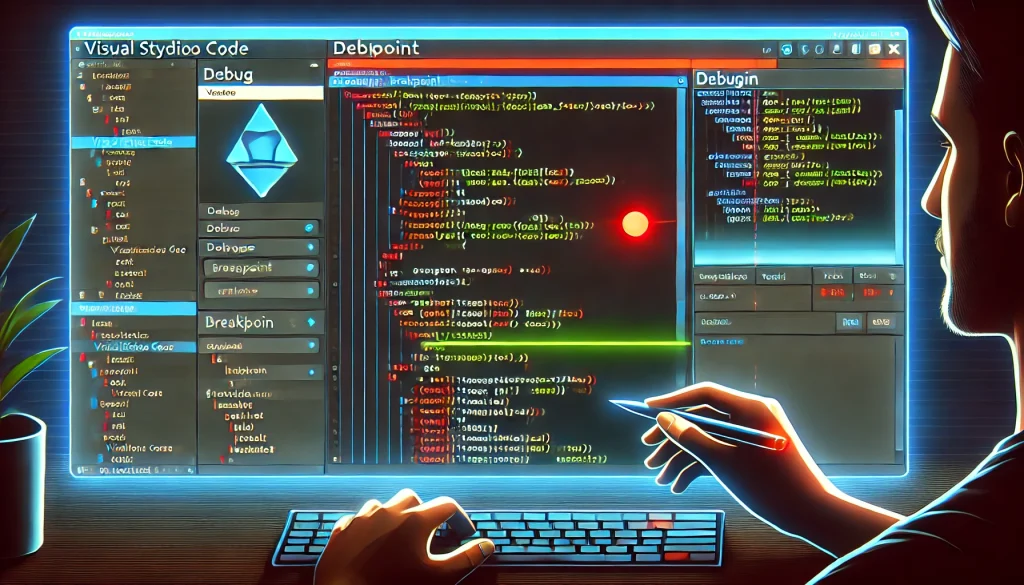
2.1 Prettier – Code Formatter
What It Does:
- Automatically formats your code (JavaScript, TypeScript, CSS, JSON, etc.) on save, ensuring a consistent style across your team.
Setup:
- Install “Prettier – Code formatter” from the VS Code Marketplace.
- Add to your
.vscode/settings.json
or user settings: jsonCopyEdit{ "editor.defaultFormatter": "esbenp.prettier-vscode", "editor.formatOnSave": true }
- Optionally define
.prettierrc
in your project for custom rules (semi, singleQuote, etc.).
Outcome: Freed from arguments over indentation or quotes, letting devs focus on logic, not style.
2.2 ESLint – Linting & Error Checking
Purpose:
- Catches syntax errors, coding style violations, or potential bugs in JavaScript/TypeScript.
Installation:
- Install the “ESLint” extension.
- Make sure your project has an
.eslintrc.js
or.eslintrc.json
config referencing relevant rules or extends (e.g.,eslint:recommended
,airbnb
).
Why: Combine with Prettier for a powerful duo: ESLint flags deeper issues or possible “footguns,” while Prettier focuses on uniform style.
2.3 Live Server
Key Benefit:
- Instantly refreshes your browser as you edit static files (HTML, CSS, JS).
- Perfect for front-end devs building quick prototypes or single-page apps that don’t need a complex local server.
How:
- Right-click an
.html
file → “Open with Live Server.” - Edits automatically reload, slashing dev time for minor style or content changes.
2.4 Debugger for Chrome / Node
Reason:
- Let’s you set breakpoints in VS Code for Node.js or front-end (Chrome-based) debugging.
- No more sprinkling
console.log
everywhere—just step through variables in real time.
Setup:
- For Node: “Debugger for Node.js” may come built-in or easily installed.
- For front-end: “Debugger for Chrome” (or similar) extension. Then create a
.vscode/launch.json
config referencing your local app URL and specifying the debug protocol.
Example:
jsonCopyEdit{
"version": "0.2.0",
"configurations": [
{
"name": "Launch Chrome against localhost",
"type": "chrome",
"request": "launch",
"url": "http://localhost:3000",
"webRoot": "${workspaceFolder}"
}
]
}
Outcome: Step through front-end code or Node scripts with watch expressions, call stacks, breakpoints—similar to a full IDE.
3. Git and Source Control
3.1 VS Code’s Built-In Git
- Source Control Tab: Displays changes, staged vs. unstaged files.
- Commit & Push: Quick commits from within the editor, plus push/pull with a single click.
Pro Tip: Use the GitLens extension for advanced blame views, commit histories, or repository insights.
3.2 Branch Management & Merging
- Branch Switching: The bottom status bar often indicates your current branch; switching is a click away.
- Pull Requests: Some dev teams integrate GitHub or GitLab PR support with optional plugins, letting you code-review directly in VS Code.
Outcome: Minimizes context switching between terminals or external GUIs, boosting dev flow.
4. Custom Snippets & Productivity Hacks
4.1 Creating Snippets
Purpose:
- Save boilerplate for repeated code, e.g.,
function
,React hooks
, or entire file templates. - Trigger them by short keywords for lightning-fast insertions.
Example (JavaScript snippet in javascript.json
under User Snippets
):
jsonCopyEdit{
"Console Log": {
"prefix": "clg",
"body": ["console.log('$1');"],
"description": "Logs output to the console"
}
}
Then typing clg + tab
expands to console.log('');
.
4.2 Shortcut Keys & Multi-Cursor
- Multi-Cursor:
Alt+Click
(Windows) orOption+Click
(Mac) to place multiple cursors. - Command Palette:
Ctrl+Shift+P
(Win) orCmd+Shift+P
(Mac) for all commands or config. - Extensions for Productivity: e.g., “Bracket Pair Colorizer” or “Peacock” for customizing your workspace theme, or “Settings Sync” to unify your environment across multiple machines.
Result: By personalizing key shortcuts, snippet usage, and color-coded settings, you create a frictionless environment that fosters creativity and speed.
5. Debugging & Testing Workflow
5.1 Node.js & Express
- Breakpoints in .js: Setting a breakpoint in the route or main app logic can clarify variable values at runtime.
- Launch Config: A
launch.json
with"program": "${workspaceFolder}/app.js"
or similar. PressF5
to start debugging.
5.2 Front-End Frameworks
- React: A live dev server run by
npm start
oryarn start
, combined with Chrome debugger extension. - Vue/Angular: Similar approach—use a local dev server, attach debugger config for your chosen browser.
Outcome: Step-by-step debugging complements ESLint and code formatting—forming a complete dev loop from code to test to commit.
6. Maintaining a Clean Workflow
- Keep It Minimal: Install only necessary extensions—too many can bloat or cause conflicts.
- Stay Updated: Let VS Code or your extensions auto-update (or at least check monthly) to fix bugs and get new features.
- Use Workspaces: For multi-module repos,
.code-workspace
files let you define separate settings or extension preferences per workspace.
Conclusion
Visual Studio Code remains a top contender for full-stack devs—blending a huge extension library with built-in Git, integrated terminals, and robust debugging. By installing Prettier for code formatting, ESLint for linting, Live Server for quick front-end previews, and a well-configured debugging environment for Node or front-end frameworks, you can drastically streamline your day-to-day. Add custom snippets for repetitive tasks, and you’ll maximize productivity. Keep your setup minimal, align with best practices in coding style and security, and watch your dev workflow flourish. Enjoy your newly curated toolkit!