Git remains the standard version control system (VCS) for modern software development. But its power can also be confusing—especially when branching strategies, rebases, and advanced merges come into play. Below are 20 essential Git commands (and some workflows) every dev team should master for a smoother collaboration experience, whether you favor GitFlow, trunk-based development, or something in between.
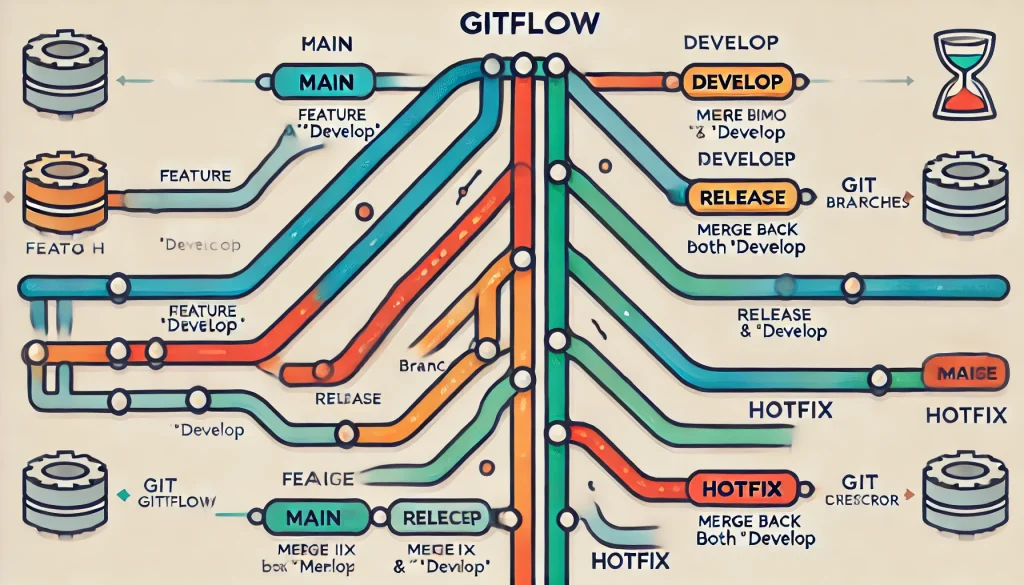
1. git init
Why It’s Important
- Starting Point: Creates a new Git repository in your current directory.
- Team Use: Usually performed once at the project’s birth, or rarely to spin off a sub-repo.
Common Syntax:
bashCopyEditgit init
Tip: If you already have code and want to track it from scratch, this is your go-to.
2. git clone
Why It’s Crucial
- Team Collaboration: Clones an existing repo from a remote server (e.g., GitHub, GitLab) to your local machine.
- Preserves History: All commits and branches are downloaded.
Common Syntax:
bashCopyEditgit clone <repository-url> [folder-name]
3. git add
What It Does
- Staging Changes: Moves modified or new files into the “staging area” so they’ll be included in the next commit.
- Selective: Use
git add -p
or specify paths to add only some changes.
Example:
bashCopyEditgit add .
# or
git add src/app.js
Tip: git add -i
/ -p
let you interactively add parts of a file, beneficial if you want to separate changes into multiple commits.
4. git commit -m "message"
Why It’s Essential
- Snapshots: Each commit is a snapshot of your project at a moment in time.
- Atomic: Aim for meaningful, self-contained commits.
Common Syntax:
bashCopyEditgit commit -m "Implement user login functionality"
Pro Tip: Use git commit --amend
to revise the last commit if you forgot some files or want to tweak the message. But be cautious if you’ve already pushed.
5. git status
Primary Purpose
- Check File States: See which files are staged, unstaged, or untracked.
- Before Committing: It’s a quick overview to confirm what’s going into your next commit.
bashCopyEditgit status
Tip: Vital for staying aware of changes when handling multiple files at once.
6. git log
Why It’s Useful
- History Browsing: Inspect commit history, authors, dates, messages.
- Customization: Use
git log --oneline --graph --decorate
for a condensed view.
Example:
bashCopyEditgit log --oneline --graph --all
Tip: Tools like tig
(text-mode interface for git) or Git GUIs can make log navigation friendlier.
7. git branch
Purpose
- Branch Management: Lists local branches or creates new ones.
- Separation of Work: Each branch can isolate features or bug fixes.
Examples:
bashCopyEdit# Create a new branch
git branch feature/cool-thing
# List branches
git branch
Tip: Many teams prefer short, descriptive branch names, e.g. feat/user-login
, fix/payment-bug
.
8. git checkout
Why It Matters
- Switching Branches: Move between different branches, or revert files to specific commits.
- Branch Creation Shortcut:
git checkout -b new-branch-name
creates and switches to a new branch in one command.
Examples:
bashCopyEdit# Switch to main branch
git checkout main
# Create and switch to new branch
git checkout -b feature/cool-thing
9. git merge
Key Use
- Combining Changes: Merges the branch you’re on with another branch’s commits.
- Workflow Approach: In GitFlow, you often merge
feature/*
branches intodevelop
orrelease
branches.
Example:
bashCopyEdit# On main branch, merge in feature branch
git checkout main
git merge feature/cool-thing
Tip: Optionally use --no-ff
to maintain a merge commit for clarity, or --ff-only
to avoid extra merges if possible.
10. git rebase
Why It’s Powerful
- Linear History: “Replay” commits from one branch on top of another, avoiding merge commits.
- Clean Commit Log: Great for smaller teams or trunk-based dev wanting fewer merge commits.
Example:
bashCopyEdit# Rebase a feature branch on top of main
git checkout feature/cool-thing
git rebase main
Warning: Avoid rebasing public branches if others rely on them—rebasing rewrites commit history.
11. git squash
(via Rebase Interactive)
Purpose
- Condense Multiple Commits: Combine commits into a single one for a more polished history.
- Example: If you made a bunch of minor fix commits, you can unify them.
Process:
bashCopyEditgit checkout feature/cool-thing
git rebase -i main
# Mark commits as "squash" or "fixup"
Tip: Ideal just before a pull request to ensure each commit is meaningful.
12. git bisect
Why It’s a Lifesaver
- Bug Hunting: Perform a binary search through commits to find which commit introduced a bug.
- Automated: Provide a script or test to define success/fail, let Git do the searching.
Example:
bashCopyEditgit bisect start
git bisect bad HEAD
git bisect good <commit-sha-known-good>
# Then you run or let Git run tests until it identifies the culprit commit
Tip: In large codebases, bisect can drastically cut down debugging time compared to manual guesswork.
13. git stash
Key Benefit
- Temporary Storage: Shelve uncommitted changes to switch branches or pull updates without losing your work.
- Restore: Bring them back later or pop them off the stash.
Examples:
bashCopyEditgit stash # Save changes
git stash apply # Reapply them
Tip: git stash pop
re-applies and removes them from stash. For multiple stashes, name them or use --index
to preserve staged files.
14. git tag
Why It’s Handy
- Marking Releases: Tag a specific commit (e.g.,
v2.0
) to track stable versions or releases. - Lightweight vs. Annotated: Annotated tags store extra info like a message or GPG signature.
Example:
bashCopyEditgit tag v2.0
git push origin --tags
Tip: Combine with CI to auto-deploy or build from tags. Also useful for quick rollbacks.
15. git push --force-with-lease
Key Use
- Rewrite History: Overwrite remote changes, typically after a local rebase or commit squash.
- Safer Force Push:
--force-with-lease
ensures no one else pushed new commits to that branch before you rewrite it.
Example:
bashCopyEditgit push origin feature/cool-thing --force-with-lease
Warning: Ensure your team consents to rewriting that branch’s shared history, or do it on personal feature branches pre-merge.
16. git pull --rebase
Why It’s Useful
- Linear Updates: Instead of merging remote changes with a new merge commit, rebase them into your local changes.
- Clean History: Minimizes “merge commits” that clutter logs.
Example:
bashCopyEditgit pull --rebase origin main
Pro Tip: Some devs configure pull.rebase = true
in Git config, to standardize rebase-based workflows.
17. GitFlow Workflow
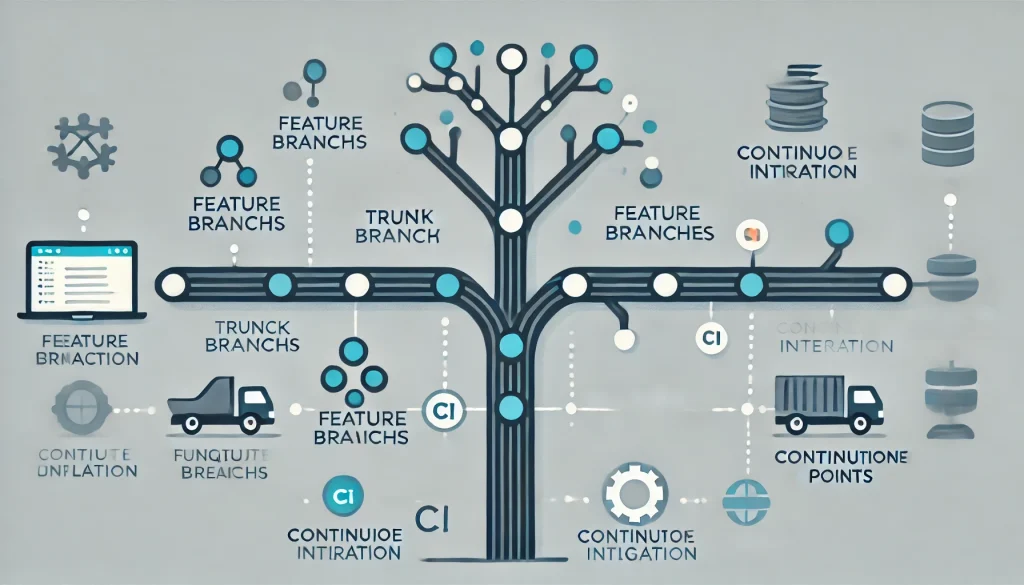
Overview
- Dedicated Branches:
main
for stable releases,develop
for integration,feature/*
for new features,release/*
andhotfix/*
branches. - Clear Process: Good for larger teams wanting structured merges.
Tip: Tools like Git Extensions or SourceTree can help visualize the branching. Perfect if your project has scheduled releases.
18. Trunk-Based Development
Overview
- Single Main Branch: Minimal branches, short-lived feature branches. Merges occur often, reducing huge merges.
- Continuous Integration: Devs commit to trunk multiple times a day, aided by robust test automation.
Advantage: Less complexity than GitFlow, fosters continuous delivery. Useful for smaller agile teams or big orgs with advanced CI gating merges.
19. Pull Request Reviews
Why It’s Vital
- Collaboration: PRs let teammates comment on changes, suggest improvements.
- Quality Control: Good PR discipline fosters better code structure, consistent style, and fewer bugs.
Tip: Incorporate status checks (CI pass, code coverage, linting) before merging. Standard PR templates help capture context for each feature or fix.
20. Code Ownership & Protected Branches
Value for Teams
- Protected Branches: Lock down
main
orrelease
to allow merges only via pull requests. - Code Owners: Some repos define owners for directories or files, requiring their approval on changes.
Outcome: This approach ensures no accidental merges to production, fosters accountability, and clarifies who must sign off for sensitive areas.
Conclusion
Whether you prefer GitFlow for predictable releases or Trunk-Based for continuous integration, mastering these 20 Git commands (and associated workflows) is essential for streamlined team collaboration.
- Basic tasks like
git add
,commit
, andpush
remain the foundation, but advanced usage—like rebasing, bisecting, and stashing—helps devs handle complex merges, isolate bugs, and maintain clean commit logs. - Pair these commands with branching strategies—GitFlow or trunk-based—and you’ll keep your team’s code consistent, auditable, and well-managed.
- Tools like pull requests, protected branches, and code owners further refine the process, ensuring each commit meets quality standards.
Takeaway: Combine the right Git commands and clear workflows, and your entire dev team gains a robust, frictionless environment for shipping features and fixing bugs with confidence.