Most developers spend significantly more time reading, navigating, and debugging code than actually writing it. That’s why productivity gains—however small—can have a huge impact on our daily workflow. While plenty of “10 tips” lists exist, many are too generic or focused on surface-level advice. In this article, we’ll explore advanced productivity techniques like keyboard-driven workflows, automating repetitive tasks, and optimizing build/test cycles. Implementing these lesser-known hacks can help you write, debug, and ship code faster.
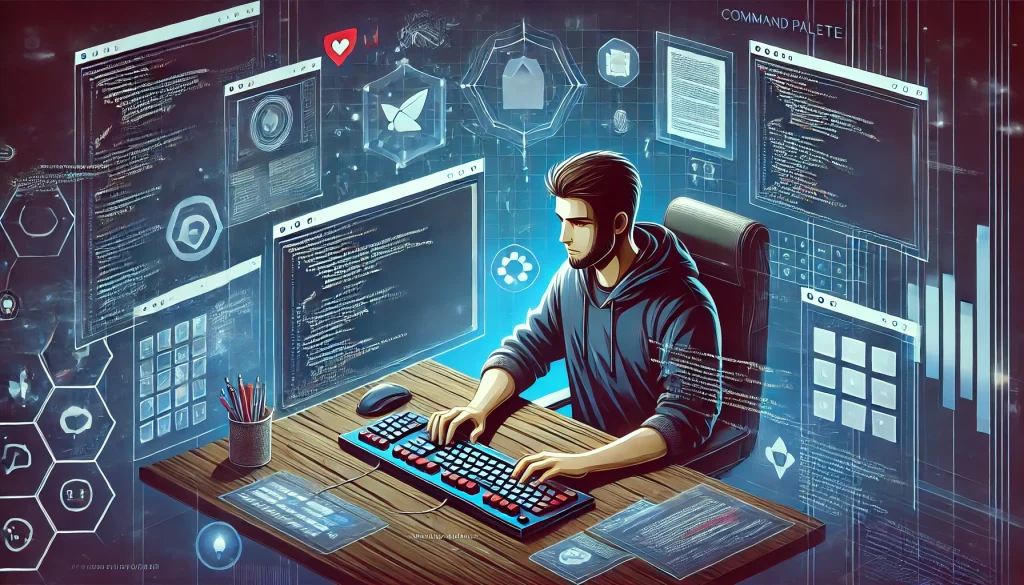
1. Embrace a Keyboard-Driven Workflow
1.1 Master Your IDE Shortcuts
Many developers already know a few shortcuts, but far fewer truly embrace a keyboard-centric approach.
- Create a Cheat Sheet: Compile essential shortcuts for actions like navigation, refactoring, and toggling tool windows.
- Practice: Try going 5–10 minutes at a time without touching your mouse; every time you reach for it, look up the keyboard method instead.
Example: IntelliJ or VS Code
- Jump to Definition:
Ctrl + B
(Windows/Linux) orCmd + B
(macOS) in IntelliJ;F12
in VS Code. - Refactor:
Ctrl + Shift + Alt + T
in IntelliJ;Ctrl + Shift + R
in VS Code.
Over time, muscle memory forms, and you’ll move around your codebase more seamlessly.
1.2 Command Palettes & Fuzzy Finders
Modern editors often include a command palette (VS Code’s Ctrl + Shift + P
or Sublime’s Cmd + Shift + P
), letting you type partial commands or filenames. This approach outperforms hunting through menus.
Tip: Tools like fzf (fuzzy finder) in your terminal can accelerate searching through files or Git commits, again minimizing or eliminating mouse usage.
2. Automate Repetitive Tasks
2.1 Scripting Common Operations
If you do something more than three times a week, consider automating it. Write small scripts or CLI tools for tasks like:
- Database migrations
- Environment setup
- Regenerating code or documentation
Use shell scripts (Bash, Zsh) or Python to orchestrate tasks, plus define common environment variables to unify your workflow.
2.2 Build & Deployment Pipelines
Extend automation to your CI/CD pipeline.
- One-Command Builds: Create a
makefile
ornpm run
script that compiles, runs tests, and deploys (where applicable). - Continuous Integration: Let a service like GitHub Actions or Jenkins run your tests on every commit. This ensures quick detection of regressions.
Bonus: If deployment is slow (exceeding a few minutes), look into incremental builds, caching, or container strategies to drastically reduce iteration time.
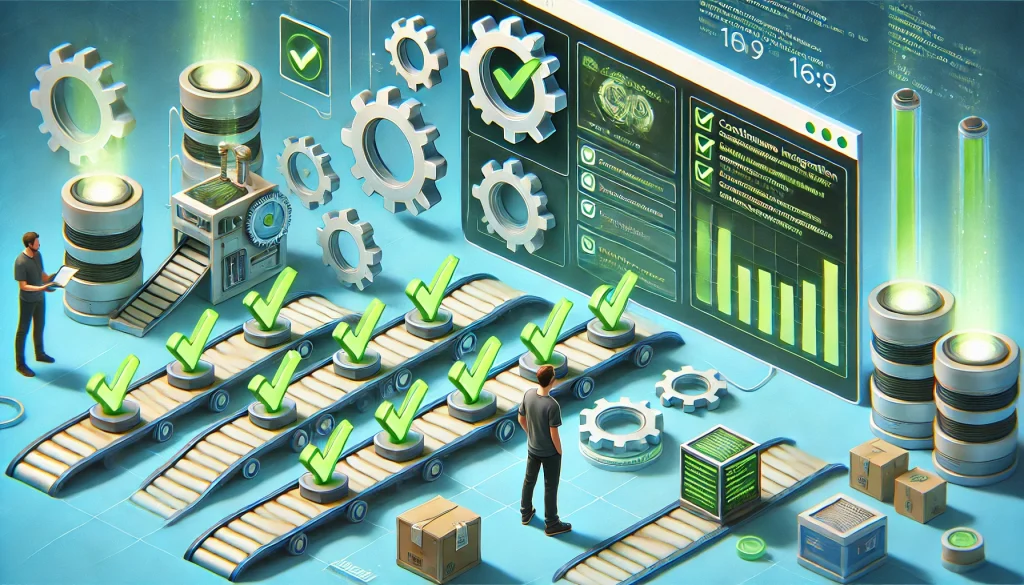
3. Optimize Build and Test Cycles
3.1 Incremental Builds
In large projects, full rebuilds can be time-consuming. Tools like Gradle, Bazel, or CMake can do incremental builds, only recompiling changed components.
Tip: For front-end projects, configure watchers (Webpack, Parcel) that rebuild only changed files. This means near-instant reloads during development.
3.2 Fast Test Feedback
- Test Watchers: Use watchers that re-run tests for only the modules you modified.
- Parallel Testing: Split your test suite across multiple CPU cores. Tools like
pytest-xdist
in Python orjest --maxWorkers
in JavaScript can cut test times significantly.
Outcome: Rapid feedback loops encourage more frequent testing and reduce the friction that often leads to ignoring tests.
4. Declutter Your Environment
4.1 Minimalist Desktop & Editor Layout
Clutter—be it on your desktop or inside your IDE—creates mental friction. A streamlined layout keeps your focus where it should be: the code.
- Close unused panels or windows
- Limit notifications: Silence random pings to stay in flow mode.
4.2 Tidy Dependencies and Tooling
Keep your environment updated. Stale or conflicting plugins can slow you down or cause weird errors.
- Cleanup Unused Plugins: If it doesn’t serve a purpose, remove it.
- Automate Tool Upgrades: For Node projects, use
npm-check-updates
; for Python, trypip-review
.
5. Advanced Debugging Shortcuts
5.1 Conditional Breakpoints
Rather than stepping through countless iterations, set conditions on breakpoints (e.g., “break when i == 100
”). This halts execution precisely when the variable or state meets specific criteria.
5.2 Watch Expressions
Leverage watch expressions in your debugger to see how key variables change over time. Tools like Chrome DevTools or IntelliJ’s debugger let you observe variable changes without constantly re-running print statements.
Example: If you suspect a data structure is being corrupted at some point, watch for changes in relevant fields across breakpoints.
6. DevOps & Collaboration Tips
6.1 Micro-Commits and Feature Branches
Commit often and keep each commit small. This practice:
- Reduces merge conflicts
- Makes code reviews easier
- Facilitates rollbacks if needed
6.2 Use Git Hooks
Automate tasks like linting, running minimal tests, or updating docs with Git pre-commit or pre-push hooks. This ensures your code passes essential checks before it ever hits the main branch.
6.3 Pair Programming and Code Reviews
Two heads are often better than one—pair programming or quick code review sessions can catch issues early and yield shared knowledge. Over time, you’ll also see best practices from your peers, accelerating your own growth.
7. Mindset: Continuous Experimentation
The most crucial productivity hack isn’t a single tool or shortcut; it’s your mindset. Keep trying new approaches or improvements to your workflow. Evaluate each idea’s impact. If it’s beneficial, incorporate it permanently. If not, discard it and move on.
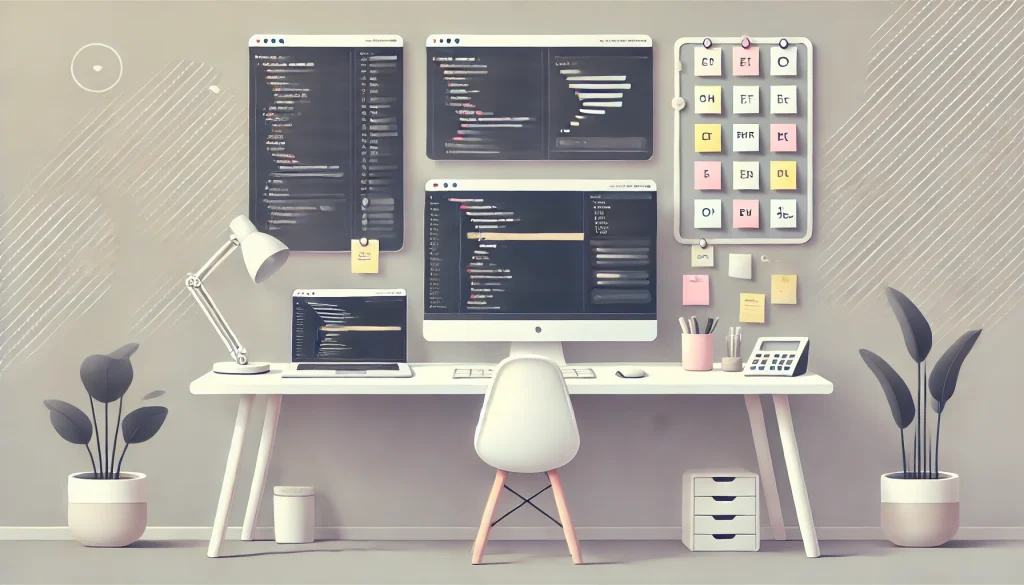
Key Takeaways:
- Keyboard-First: Mastering shortcuts and fuzzy finders significantly reduces context-switching overhead.
- Automation: Scripting, robust CI/CD, and incremental builds slash repetitive tasks and keep you in flow.
- Optimized Testing: Quick test feedback encourages quality code and frequent iteration.
- Tidy Environment: Minimal distractions lead to deeper focus.
- Continuous Tweaks: Regularly refine your process, adopting or discarding methods as needed.
By implementing these advanced productivity hacks, you’ll transform your daily workflow from a series of small frustrations to a streamlined pipeline, letting you spend more energy on actual problem-solving.