Concurrency has become essential in modern programming, especially with multicore processors fueling performance gains. However, multithreaded code introduces complexities: race conditions, deadlocks, and mysterious data corruptions can be notoriously hard to reproduce and fix. Despite growing developer interest, resources remain scattered, and many solutions are case-specific.
This article aims to fill the gap, offering a structured approach to detecting, diagnosing, and resolving concurrency bugs in multithreaded applications.
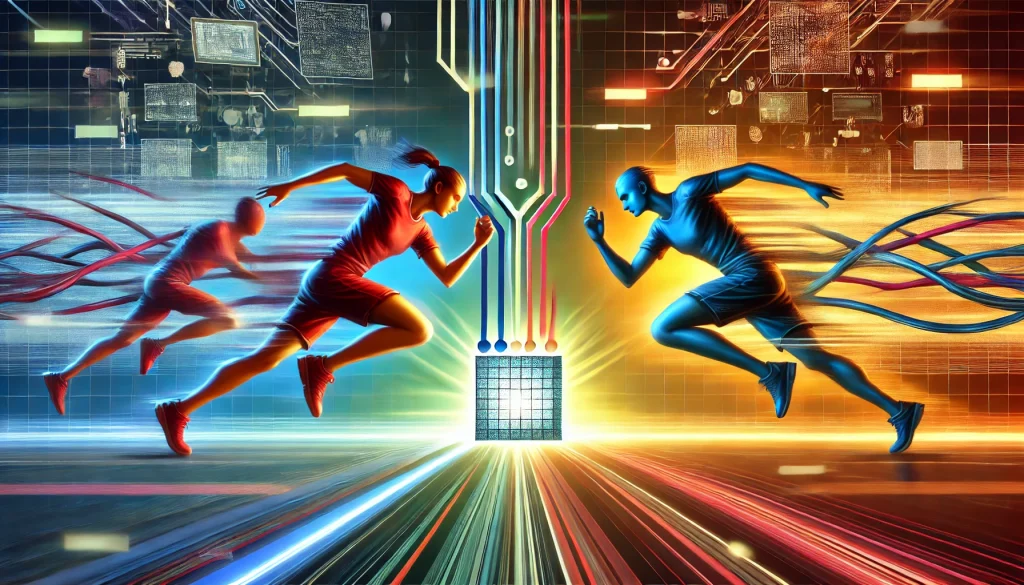
1. Why Concurrency Bugs Are So Hard
1.1 Race Conditions in a Nutshell
A race condition happens when multiple threads access or modify shared data concurrently, and the final outcome depends on timing or thread interleavings. Such interleavings can be incredibly difficult to anticipate or replicate consistently. A program may run correctly thousands of times before a subtle timing shift triggers the bug.
1.2 Deadlocks and Livelocks
A deadlock occurs when two (or more) threads are blocked, each waiting for resources locked by the other. Neither can proceed, and the application becomes stuck. Livelocks are similar, except the system is still active but continuously failing to progress.
1.3 Heisenbugs
“Heisenbug” is a playful term for concurrency defects that seem to disappear when you add logging or step through the debugger. This phenomenon occurs because instrumentation or breakpoints change the timing of thread scheduling—masking the original bug.
2. Setting the Stage: Concurrency Fundamentals
2.1 The Shared State Problem
When multiple threads access the same memory location without proper synchronization, unpredictable results can occur. Common synchronization mechanisms include:
- Mutexes (locks): Enforce mutual exclusion on critical sections.
- Semaphores: Control access to a finite resource pool.
- Atomic Operations: Provide lock-free methods for incrementing counters or comparing-and-swapping values.
- Read-Write Locks: Differentiate between multiple readers and exclusive writers.
2.2 Memory Models and Ordering
Modern CPUs and programming languages define memory models that describe how operations on different threads are ordered (or not). For instance, reordering optimizations can break assumptions if code lacks the right fences or volatile
semantics. Understanding the relevant memory model (e.g., C++11, Java’s JMM) is crucial.
2.3 Thread Scheduling
Operating systems typically use preemptive scheduling, deciding which thread runs at any given moment. This can lead to near-infinite permutations of how threads interleave, especially as core counts increase. Thus, concurrency bugs can remain dormant if an unlucky schedule never emerges in testing.
3. Common Concurrency Bugs to Watch Out For
- Data Races
- Multiple threads read and write the same data without synchronization.
- Example: Two threads updating a shared counter but skipping an atomic operation or lock.
- Deadlocks
- Thread A holds Lock X, needs Lock Y. Thread B holds Lock Y, needs Lock X. Both wait forever.
- Typically occurs when locks aren’t acquired or released in a consistent global order.
- Resource Starvation
- A lower-priority thread never gets CPU time because higher-priority threads monopolize resources.
- Can manifest in real-time systems or specialized scheduling scenarios.
- Priority Inversion
- A low-priority thread holds a lock that a high-priority thread needs, but a medium-priority thread continuously runs, blocking the low-priority thread from releasing the lock.
- Without priority inheritance mechanisms, this can stall critical tasks.
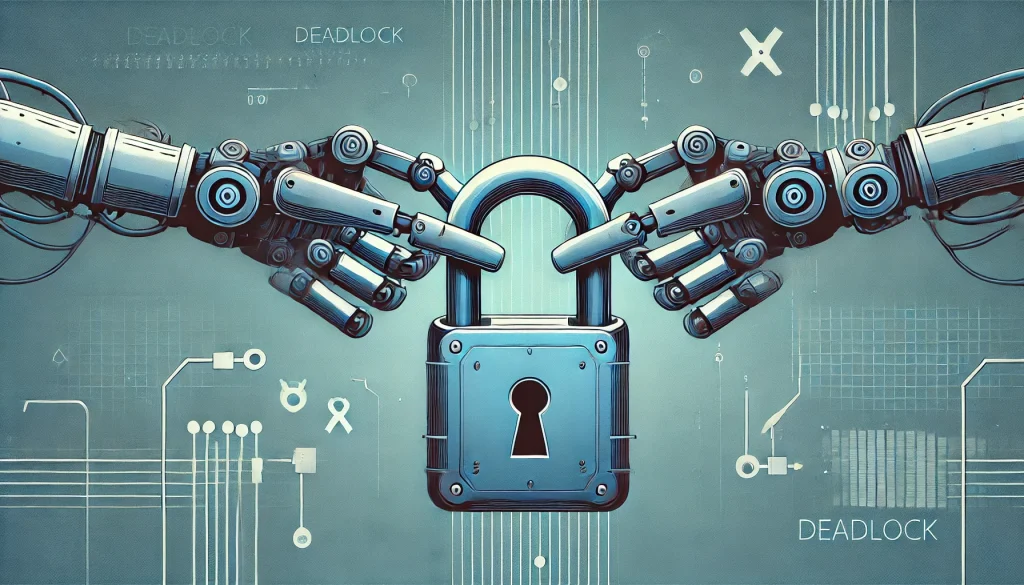
4. Debugging Strategies: Detection & Diagnosis
4.1 Logging and Tracing
- Thread-Tagged Logs
- Label log statements with thread IDs to track interleavings.
- Tools like Log4j (Java) or spdlog (C++) can include a thread context in each log entry.
- Tracing Frameworks
- Tools such as LTTng (Linux), ETW (Windows), or Trace Compass for analyzing system events.
- Let you visualize how threads schedule and when locks are acquired or released.
Tip: Logging can inadvertently change timing. So be minimal or consistent—excessive logs might hide the race condition.
4.2 Specialized Debuggers and Tools
- Thread Sanitizer (TSan)
- Available in Clang, GCC, and sometimes integrated in IDEs.
- Dynamically detects data races and synchronization issues with minimal code changes.
- Valgrind Helgrind/DRD (Linux)
- Helgrind or DRD detect race conditions, lock usage errors, etc.
- Effective for debugging but can slow execution significantly.
- Visual Studio Parallel Stacks/Tasks
- For Windows devs, Visual Studio includes parallel debugging views to track threads and tasks.
- Helps identify deadlocks or unresponsive threads.
- Java Thread Dump & VisualVM
- In Java, a
jstack
thread dump or using VisualVM can show thread states (RUNNABLE, BLOCKED, WAITING). - Useful for diagnosing deadlocks (
Found one Java-level deadlock:
messages).
- In Java, a
4.3 Replaying or Deterministic Execution
Tools like rr (on Linux) record a program’s execution so you can deterministically replay it in a debugger. Although overhead is high, it’s priceless for reproducing ephemeral concurrency bugs exactly as they occurred.
5. Advanced Debugging Techniques
5.1 Delay Injection & Stress Tests
- Random Sleep or Artificial Delays: Insert random sleeps or yield calls within critical sections to force unusual interleavings.
- Chaos Engineering: Induce resource constraints or partial failures to see if concurrency code gracefully handles them.
5.2 Lock Order Verification
- Lock Hierarchies: Always acquire locks in a consistent order (e.g., alphabetical by lock name).
- Runtime Checks: Some libraries provide lock order checkers that detect potential deadlock cycles if multiple locks are held simultaneously.
5.3 Model Checking and Formal Methods
- TLA+ or SPIN: Tools to model concurrency logic and exhaustively check for safety or liveness violations.
- State Space Explosion: The downside is scale—these methods can be complex, but they’re powerful for critical systems with strict correctness requirements.
6. Best Practices to Prevent Concurrency Bugs
- Immutable Data
- Keep data structures immutable whenever possible. Concurrency issues shrink when objects can’t be changed.
- In functional languages (e.g., Scala, F#), immutability is encouraged by default.
- Minimize Shared State
- Pass messages or events instead of direct data sharing (e.g., actor-based models).
- If shared memory is unavoidable, use appropriate synchronization or safe concurrency patterns.
- Use Thread-Safe Libraries
- Lean on proven concurrency wrappers (ConcurrentHashMap in Java, concurrent containers in C++17+).
- Understand their usage patterns and limitations.
- Testing in a Realistic Environment
- Run load tests on multicore machines, randomizing scheduling.
- Cloud-based tests with ephemeral environments can surface concurrency issues not seen locally.
- Review and Pair Programming
- Code reviews focusing on concurrency can catch subtle mistakes in lock usage or data sharing.
- Pair or mob programming encourages real-time checks: “Are we sure we’re not referencing shared data here?”
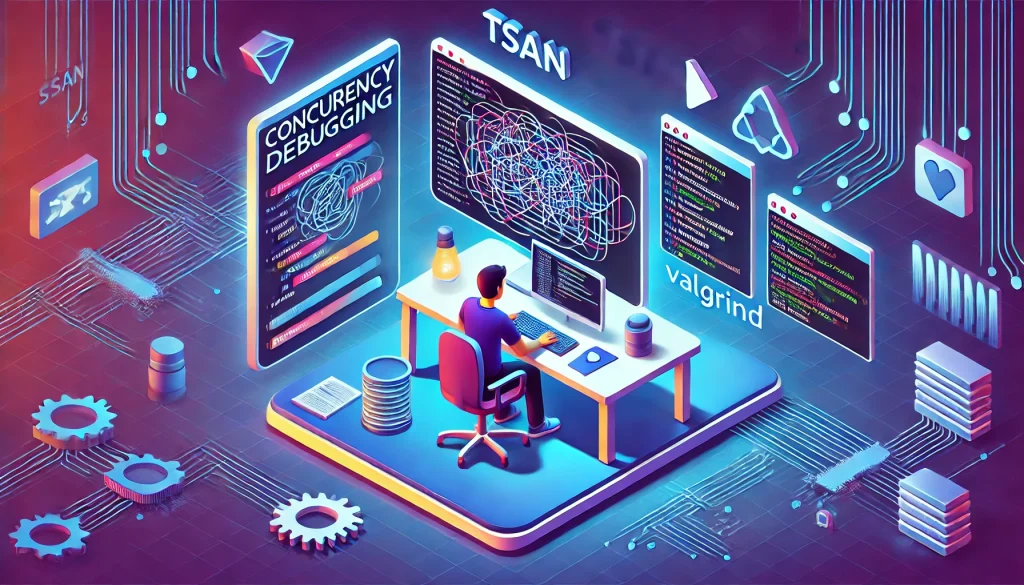
7. Conclusion
Debugging concurrency in multithreaded systems is challenging because the slightest timing difference can mask or expose a bug. However, with the right tools—such as TSan, Valgrind Helgrind, or advanced debuggers—and techniques—like logging, formal verification, or chaos testing—you can systematically pinpoint and resolve elusive race conditions, deadlocks, and other concurrency pitfalls.
Key Takeaways:
- Start with a solid concurrency design: limit shared state, define lock orders, and use proven libraries.
- Use specialized debugging tools (sanitizers, thread analyzers) to catch hidden race conditions.
- Reproduce concurrency issues by injecting random delays or employing deterministic replay.
- Keep concurrency reviews front-and-center in your development cycle—don’t wait for production crashes to discover hidden heisenbugs.
By applying these strategies, you’ll be better equipped to tackle concurrency bugs head-on and build robust, thread-safe applications in a world where multicore processing has become the norm.