Performance matters in almost every software domain, from web apps to embedded systems. Yet many developers struggle with where and how to begin profiling their code. They either optimize too early, risking wasted effort, or too late, leading to slowdowns in production. This guide will walk you through essential profiling steps, interpreting performance data, and best practices to ensure you optimize effectively.
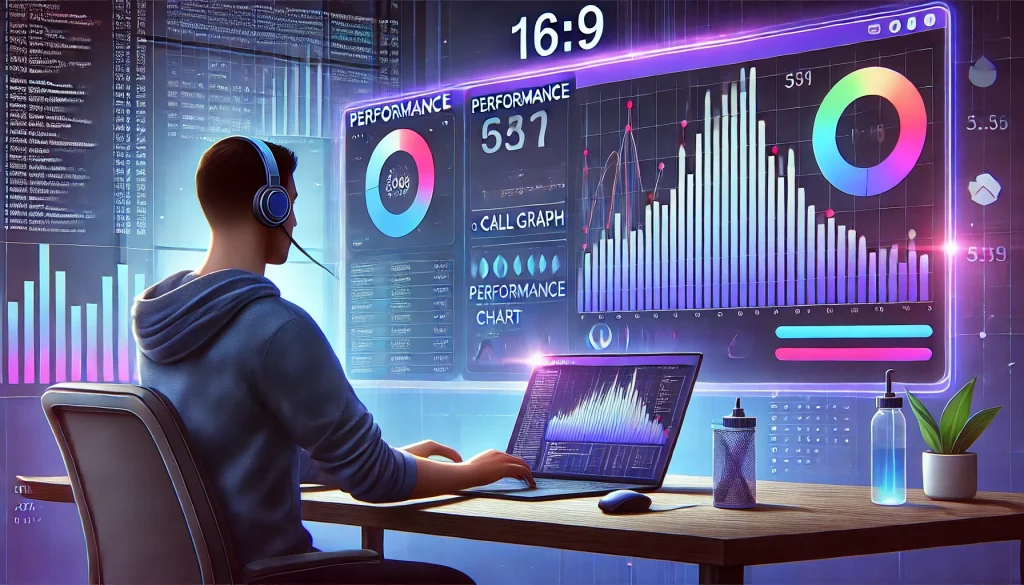
1. Why Profiling Matters
1.1 Understanding Bottlenecks
Without concrete data, you might guess at performance issues—often incorrectly. For instance, you could spend hours refining a small function that runs infrequently, while a hidden database call accounts for 80% of your runtime. Proper profiling pinpoints real bottlenecks so that your optimization efforts yield tangible gains.
1.2 Avoiding Premature Optimization
“Premature optimization is the root of all evil,” as Donald Knuth once said. Constant micro-tweaks often produce minimal benefits but increase code complexity. Profiling ensures you only optimize hot spots—the code paths that run frequently or handle large data volumes.
1.3 Real-World Impact
Whether you’re building mobile apps that need snappy responsiveness or enterprise servers that must handle millions of requests, performance can define user satisfaction and operational costs. By gathering metrics early and fixing bottlenecks, you’ll craft solutions that run efficiently at scale.
2. Profiling Fundamentals
2.1 Types of Profilers
- Sampling Profilers
- Periodically check which function is running.
- Lightweight, often the default approach in modern IDEs (e.g., Visual Studio, IntelliJ).
- Example: Linux’s
perf
, macOS’s Instruments, or Windows Performance Analyzer.
- Instrumenting Profilers
- Insert hooks or rewrite code to track entry/exit times for functions.
- Provides detailed call counts but can add overhead, potentially altering performance characteristics.
- Example: Intel VTune, Java Flight Recorder, or gprof (for C/C++).
- Event Tracing Profilers
- Log system- or runtime-level events (context switches, memory allocations).
- Tools like ETW (Windows), LTTng (Linux) help you see broader system interactions.
2.2 Key Metrics & Terminology
- CPU Time: The amount of active CPU usage by a function or thread.
- Wall-Clock Time: Real elapsed time, including waiting on I/O or synchronization.
- Call Graph: Visualization of how functions call each other.
- Hot Path: The path of execution that consumes the most CPU or runtime.
3. Getting Started with Profiling
3.1 Define Performance Goals
Before you open a profiler, clarify what you need to improve. For example:
- Reducing page load time from 3s to under 1s.
- Handling 10,000 requests per second on a REST API.
- Cutting memory use by 30% to avoid out-of-memory errors on small devices.
Clear goals help you track actual progress rather than chasing random speedups.
3.2 Choose the Right Environment
Profile code in an environment that mimics production conditions as closely as possible. Debug builds or running in a VM with fewer cores than production can yield misleading data.
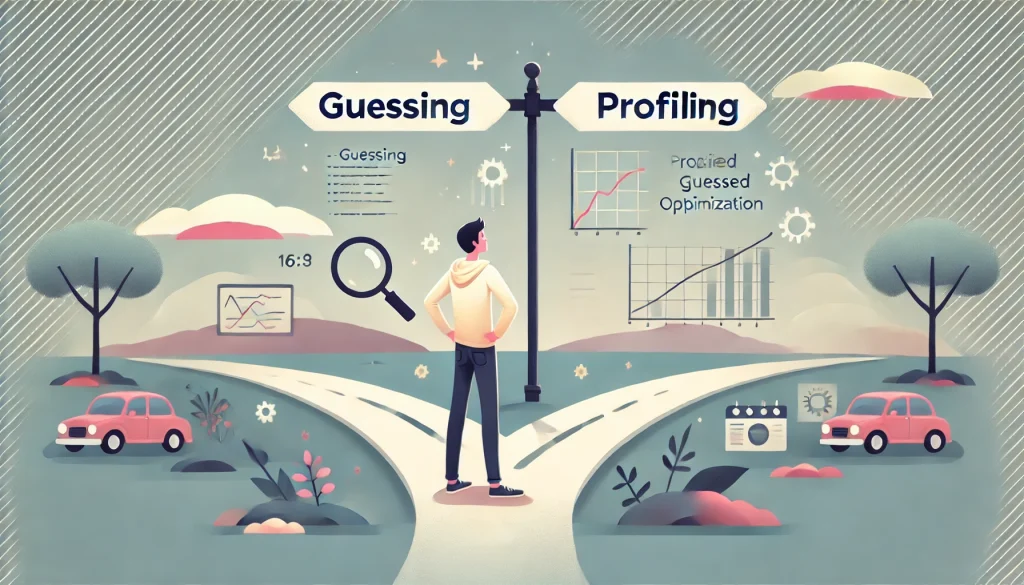
3.3 Basic Profiling Steps
- Warm Up: For languages like Java or .NET, JIT compilation affects initial runs. Let the app run a bit.
- Gather Baseline: Run the profiler without changes. Note baseline CPU usage, memory usage, and response times.
- Identify Hot Spots: Use call graphs or CPU usage charts to see which function or method consumes the most time.
- Analyze: Cross-reference with logs or domain knowledge. Is the function called too often? Or is it heavy due to inefficient algorithms?
4. Interpreting Profiling Data
4.1 CPU vs. I/O Bottlenecks
- CPU-Bound: The code uses most of the CPU time. Possibly a complex algorithm or tight loop.
- I/O-Bound: Spends time waiting for disk, network, or database. Fixing CPU loops won’t help; optimizing queries or using asynchronous I/O might.
4.2 Memory Bottlenecks
Excessive garbage collection or high allocation rates can slow down applications. Look for:
- Frequent short-lived allocations in loops.
- Spikes in GC activity (for managed languages) at peak load.
4.3 Hot Call Paths & Inlining
Profilers often highlight “hot call paths,” the chain of function calls that lead to high CPU usage. Understanding whether inlining or flattening certain calls can help is crucial, especially in C++ or Java, where the compiler/JIT decides which functions to inline.
5. Optimization Techniques
5.1 Algorithmic Improvements
Sometimes a better algorithm outperforms micro-optimizations. For example, using a hash map instead of a linear search can produce exponential speedups. Revisit your data structures—O(n^2) solutions might be fine for small inputs, but break down at scale.
5.2 Parallelization and Concurrency
If CPU usage is high and your system has idle cores, consider parallel processing. However, concurrency adds complexity and can degrade performance if not managed carefully (due to synchronization overhead).
5.3 Micro-Optimizations
- Loop Unrolling: Minimizes loop overhead, though modern compilers can do this automatically.
- Reducing Function Calls: In performance-critical inner loops, cutting down function call overhead helps.
- Cache Utilization: For languages like C/C++, ensure data structures are laid out in memory to improve cache locality.
5.4 Caching Results
If a function repeatedly computes the same results with identical inputs, caching can eliminate redundant computations. But watch out for memory usage and potential stale data issues—memoization is often best for pure functions with no side effects.
6. Avoiding Premature Optimization Pitfalls
- Measure, Don’t Guess
- Always use data from a profiler or performance tests before changing code.
- Educated guesses can still be off by orders of magnitude.
- Keep Code Maintainable
- Too many micro-optimizations can reduce readability. Balance performance with clarity.
- Add inline comments explaining why a seemingly odd approach was chosen for performance reasons.
- Repeat the Profiling Cycle
- After each optimization, re-profile to ensure you’re still targeting the correct bottleneck and not introducing new ones.
7. Case Study: Example Flow
Suppose you have a web API that’s slow under high traffic:
- Baseline Profiling: Tools like VisualVM (Java), dotTrace (.NET), or perf (C/C++ on Linux). Identify your service spending 50% CPU time in JSON serialization.
- Analysis: JSON library is used in a naive loop, allocating many objects.
- Optimization: Switch to a more efficient serialization library or reduce unnecessary field serialization.
- Second Profiling: CPU usage for serialization drops from 50% to 10%. The next bottleneck might be the database calls—repeat the cycle.
8. Final Thoughts
Profiling is both an art and a science—collecting raw data is mechanical, but interpreting it and designing appropriate optimizations is more nuanced. The payoff for a structured approach is huge: you avoid guesswork, solve the right performance problems, and keep your codebase healthy.
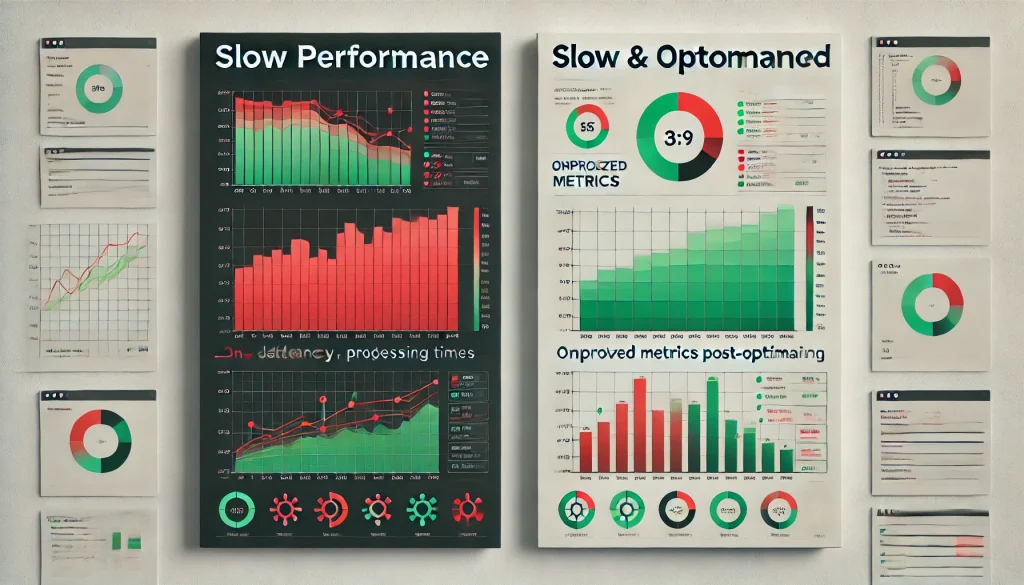
Key Takeaways:
- Set Clear Targets: Know your performance goals.
- Pick the Right Tool: Sampling, instrumentation, or event-tracing depends on your environment.
- Interpret Data Objectively: Focus on hot paths or heavily used resources.
- Optimize Iteratively: Check improvements after each change.
- Aim for Maintainable Solutions: Don’t bury your colleagues in obfuscated “optimizations.”
By mastering profiling tools and techniques, you’ll confidently address real performance bottlenecks—ensuring your code runs efficiently where it matters most.