Introduction
Cross-Origin Resource Sharing (CORS) errors are a frequent stumbling block for web developers. These errors typically occur when your front-end application and back-end service are on different domains or ports. While the underlying concept is straightforward—protecting users from malicious requests—debugging and configuring CORS can still be confusing.
This guide will help you understand:
- What the same-origin policy is and why CORS errors happen.
- Common server configurations to enable CORS.
- Practical examples in Express.js and Nginx.
By the end, you will know how to prevent and troubleshoot the most common CORS issues, ensuring smoother communication between your front-end and back-end.
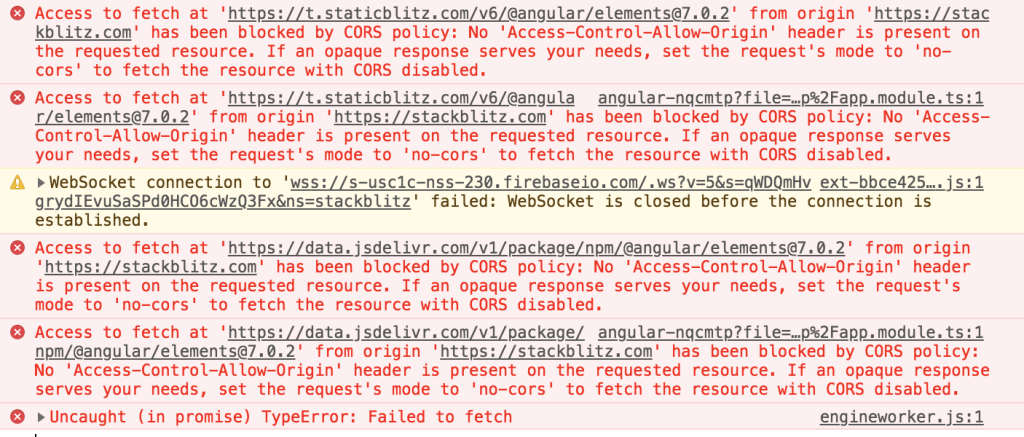
1. Understanding the Same-Origin Policy
1.1 What is the Same-Origin Policy?
The same-origin policy is a security mechanism used by browsers. It restricts web pages from making requests to a different origin (domain, protocol, or port) than the one it was served from. This policy helps prevent malicious scripts from hijacking user data on different websites.
Example:
- Origin A: https://example.com
- Origin B: https://api.example.com
These are different origins because the subdomains differ (example.com
vs. api.example.com
). A standard fetch request or XHR from Origin A to Origin B may trigger a CORS error if B does not allow cross-origin requests from A.
1.2 How Does CORS Fit In?
CORS (Cross-Origin Resource Sharing) is a way for servers to say, “I’ll accept requests from this external origin.” Through specific headers, the server grants permission to cross-origin clients. When a request lacks these headers or has them misconfigured, the browser blocks it.
2. Typical CORS Errors and Their Causes
- Missing CORS Headers: The server does not include the
Access-Control-Allow-Origin
header. - Wildcard vs. Specific Origins: Using
*
might be blocked in certain requests, especially for credentials-based requests (cookies, HTTP auth). - Preflight Failures: Complex requests (e.g.,
PUT
orDELETE
) trigger a preflight check. If the server does not respond correctly to OPTIONS, the actual request is never sent. - Credentials Errors: If you send cookies or other credentials but do not enable
Access-Control-Allow-Credentials
, the request fails.
Sample Error Message in Console:
csharpCopyEditAccess to fetch at 'https://api.example.com/data' from origin 'https://example.com'
has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present...
3. Configuring CORS in Express.js
Express.js is a popular Node.js framework for building APIs. Below is a quick overview of how to fix CORS issues in an Express app.
3.1 Using the cors Package
One of the easiest ways to handle CORS is to use the official cors
package. Install it via npm
or yarn
, then add it to your Express server:
bashCopyEditnpm install cors
Then, in your Express application:
jsCopyEditconst express = require('express');
const cors = require('cors');
const app = express();
// Basic usage (allows all origins)
app.use(cors());
// For more advanced usage, specify options:
const corsOptions = {
origin: 'https://example.com', // or an array of allowed origins
methods: 'GET,HEAD,PUT,PATCH,POST,DELETE',
credentials: true, // enable set cookie
optionsSuccessStatus: 204
};
app.use(cors(corsOptions));
app.get('/data', (req, res) => {
res.json({ message: 'Hello from Express!' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Key Points:
origin
can be a string or array. Use*
for any origin, but be cautious with security.credentials: true
allows cookies or HTTP auth to be sent.methods
can restrict which HTTP verbs are allowed.
3.2 Handling Preflight Requests
Express automatically handles OPTIONS requests when using the cors
middleware. If you prefer manual handling, ensure you respond with the correct headers for all required methods.
4. Configuring CORS in Nginx
Many developers use Nginx as a reverse proxy or as the primary server. Below is a simple snippet for enabling CORS in your server
block.
nginxCopyEditserver {
listen 80;
server_name api.example.com;
location / {
add_header 'Access-Control-Allow-Origin' 'https://example.com' always;
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS, DELETE, PUT';
add_header 'Access-Control-Allow-Headers' 'Content-Type, Authorization';
add_header 'Access-Control-Allow-Credentials' 'true';
if ($request_method = OPTIONS ) {
add_header 'Access-Control-Max-Age' 1728000;
add_header 'Content-Type' 'text/plain charset=UTF-8';
add_header 'Content-Length' 0;
return 204;
}
proxy_pass http://localhost:5000;
}
}
Explanation:
Access-Control-Allow-Origin
: Set this to your front-end origin or use*
for any.Access-Control-Allow-Methods
: Lists which HTTP verbs (e.g., GET, POST) are allowed.Access-Control-Allow-Headers
: Must match any custom headers the browser sends.add_header 'Access-Control-Allow-Credentials' 'true'
: Needed if cookies or session tokens should be exchanged.- Preflight Handling:
if ($request_method = OPTIONS)
ensures that Nginx returns status 204 with the correct headers for preflight requests.
5. Common Pitfalls and How to Avoid Them
- Forgetting Credentials
- If you send cookies but do not enable
Access-Control-Allow-Credentials
, requests fail silently. - Similarly, if you enable credentials,
Access-Control-Allow-Origin
cannot be*
; it must be explicit.
- If you send cookies but do not enable
- Specifying Wrong Headers
- If your front-end sends custom headers (e.g.,
X-Auth-Token
), add them toAccess-Control-Allow-Headers
.
- If your front-end sends custom headers (e.g.,
- Mixing HTTP and HTTPS
- Modern browsers may block content if you mix secure and non-secure origins.
- Overly Permissive Config
- Using
*
might expose your API to unauthorized domains. Consider dynamic whitelisting or checking theOrigin
header.
- Using
6. Step-by-Step Troubleshooting Guide
- Check Console or Network Tab
- Look for the exact error message. Often, it indicates which header is missing or if an OPTIONS request failed.
- Review Server Logs
- Confirm that your server is returning the correct
Access-Control-*
headers.
- Confirm that your server is returning the correct
- Test Preflight
- Manually run an OPTIONS request (using Postman or curl) to see if you receive the right response.
- Avoid Mixed Protocols
- If your client is on HTTPS, your server should also be on HTTPS to prevent browser blocks.
- Use Tools and Debugging
- Tools like the browser’s DevTools or Postman can isolate if the problem is with the server or a misconfigured header.
Conclusion
CORS issues can derail an application’s user experience when front-end and back-end services reside on different domains. By understanding the same-origin policy and configuring CORS headers properly, you can ensure your web app communicates seamlessly across origins.
Key Takeaways:
- Enable CORS using headers like
Access-Control-Allow-Origin
andAccess-Control-Allow-Methods
. - Choose Specific Origins rather than
*
if you need credentials and improved security. - Test Preflight Requests manually or with a debugging tool to confirm correct server responses.
- Remain Vigilant about changing domain names, custom headers, or protocol shifts that can break your existing config.
By applying these best practices in Express.js, Nginx, or any other server environment, you will reduce the likelihood of CORS headaches and deliver a smoother client-server experience.