Introduction
NullPointerExceptions (NPEs) are some of the most common runtime errors Java developers face. They typically appear when your code tries to access a method or variable on a null reference, causing the program to crash. Although NullPointerExceptions are straightforward to explain, they can be tricky to debug and prevent.
In this guide, you will learn:
- Why NullPointerExceptions occur.
- Common scenarios where they appear.
- Techniques to fix and avoid them.
- Best practices for handling null in Java.
By the end, you will have the knowledge to write more robust and reliable code.
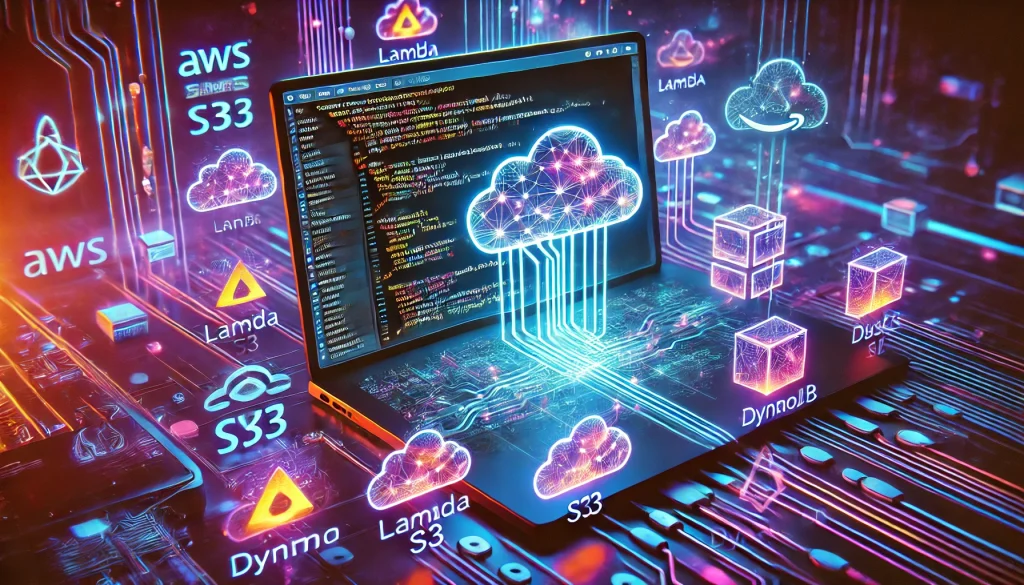
What is a NullPointerException?
A NullPointerException occurs when your Java code attempts to use an object reference that has not been initialized (i.e., it is null). Specifically, this error is thrown if you try to:
- Call an instance method on a null reference.
- Access a field (variable) of a null reference.
- Use a null reference in place of an object (e.g., in array access).
Since Java references can point to no object, your code must confirm an object exists before calling its methods or reading its fields.
Example:
javaCopyEditString name = null;
System.out.println(name.length()); // Throws NullPointerException
In this snippet, the name
reference is null, so the length()
method cannot be called.
Common Causes of NullPointerExceptions
1. Uninitialized Variables
Sometimes, you may declare a variable without assigning it a value. When you try to use that variable, it might still be null.
javaCopyEditpublic class User {
String username; // uninitialized, defaults to null
public User() {
// 'username' is not assigned
}
public void printUsername() {
System.out.println(username.toUpperCase()); // NPE if username is null
}
}
2. Return Values that are Null
Method calls can also return null, especially if an item is missing or an error occurs. If you forget to check the returned value, you risk triggering an NPE.
javaCopyEditString getCity() {
return null; // Possibly due to a missing record
}
System.out.println(getCity().toLowerCase()); // NPE if getCity() returns null
3. Chaining Methods
Chained calls can look neat, yet they can hide potential NPEs if any method in the chain returns null.
javaCopyEdituser.getAddress().getCity().toUpperCase();
// If user.getAddress() is null, an NPE occurs before getCity() is called.
4. Improper Use of Static Fields
Storing a null reference in a static field can lead to unexpected errors when multiple classes access it. This can happen if the static field is never set or is reset to null incorrectly.
Best Practices to Avoid NPEs
1. Initialize Variables Properly
Assign default values to fields and local variables as soon as possible. If you rely on constructor parameters, ensure they are not null or handle them with checks.
javaCopyEditpublic User(String username) {
if (username == null) {
this.username = "Guest"; // fallback
} else {
this.username = username;
}
}
2. Use Defensive Checks
Before calling methods or accessing fields, check if the reference is null. This approach, often called defensive coding, can prevent crashes.
javaCopyEditif (user != null && user.getAddress() != null) {
System.out.println(user.getAddress().getCity());
}
3. Embrace Optional (Java 8+)
Java’s Optional<T>
can encapsulate values that might be null. Instead of returning null, return an Optional
object to signal that the value could be absent.
javaCopyEditpublic Optional<String> getCity() {
// return Optional.of(city) if not null
// else Optional.empty()
}
getCity().ifPresent(city -> System.out.println(city.toLowerCase()));
Using Optional
clarifies which methods may return null-like states and encourages safer handling.
4. Use Annotations
Libraries like Lombok or Checker Framework include annotations (e.g., @NonNull
, @Nullable
) that indicate whether a parameter or return value can be null. Static analysis tools then warn you if you misuse these methods.
javaCopyEdit@NonNull
private String lastName;
5. Avoid Chaining Methods Without Checks
Method chaining is concise, yet each method call adds risk if any of them returns null. Use optional checks or break down the chain into smaller steps that check for null values.
Troubleshooting NullPointerExceptions
- Check the Stack Trace: The error log usually pinpoints the exact line where the NPE happened. Identify the variable or method call on that line.
- Trace Variable Flow: Look at where the variable is supposed to be set. Did you forget to initialize it or handle a missing data condition?
- Use a Debugger: Inspect variable states at runtime. Confirm if any references remain null when they shouldn’t.
- Add Defensive Checks: If the reference can sometimes be null, handle it gracefully with an if-statement or
Optional
.
Example: Fixing an NPE Step-by-Step
Suppose you have this code:
javaCopyEditpublic String getUserCity(User user) {
return user.getAddress().getCity();
}
An NPE occurs if user
is null or if getAddress()
returns null. Here is one way to fix it:
javaCopyEditpublic String getUserCity(User user) {
if (user == null || user.getAddress() == null) {
return "Unknown City";
}
return user.getAddress().getCity();
}
This solution checks for null references and provides a default string when data is missing.
Conclusion
NullPointerExceptions in Java often stem from uninitialized variables, unexpected null returns, or overlooked edge cases. By using defensive checks, initializing variables, and adopting modern Java features like Optional, you can drastically reduce the risk of NPEs. Furthermore, tools like static analyzers and annotations offer early warnings when code paths may result in null references.
Key Takeaways
- Always initialize variables, either at declaration or in constructors.
- Embrace
Optional
for methods that may return a missing or invalid value. - Use defensive coding to handle uncertain data.
- Review stack traces and method calls carefully to spot potential null references.
By following these best practices, you can prevent most NullPointerExceptions and write more resilient Java applications.